Handling Errors in Golang: Techniques and Patterns
Learn different error handling techniques in Go, including checking for errors, panic and recover, error wrapping, and custom error types. Write more reliable and resilient code in Go.
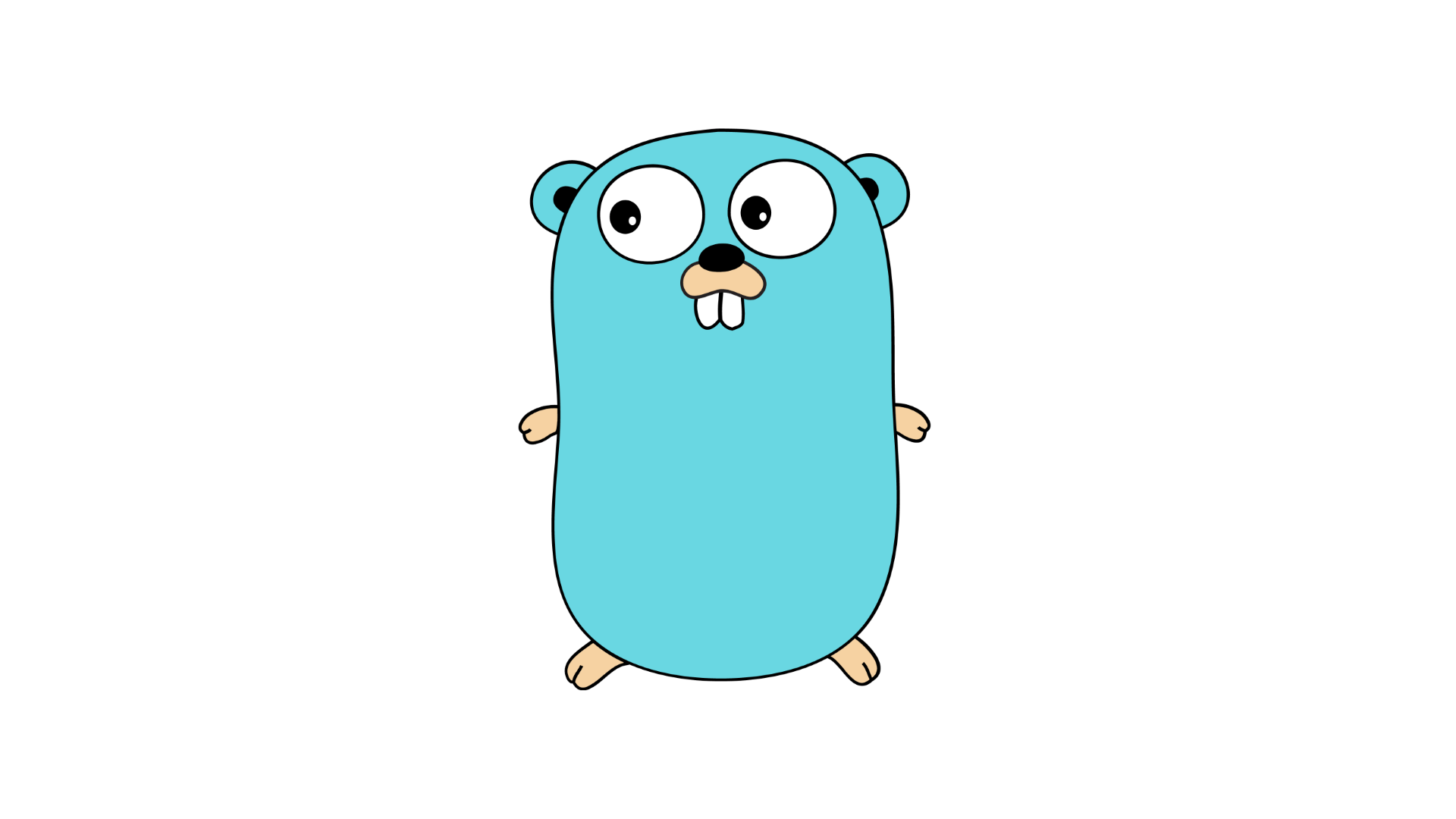
Introduction
Error handling is an essential aspect of any programming language, and Go (Golang) provides developers with powerful tools and techniques to handle errors effectively. In this blog post, we will explore different error handling techniques and patterns in Go, helping you write more robust and reliable code.
Error Handling in Golang
Go uses a unique approach to error handling that sets it apart from other languages. Instead of using exceptions, Go embraces a more explicit error handling approach using "errors" as a built-in type. The error type in Go is an interface type that provides just one method, Error(), which returns a string describing the error.
When a function or method has a return value of type "error", it means that it can return an error as one of its results. The caller of such a function is responsible for checking the returned error value and deciding how to handle it.
1. Checking for Errors
One common error handling technique in Go is to check for errors explicitly after making a function call. This technique ensures that you don't ignore any potential errors and allows you to take appropriate action based on the error.
Here's an example of how to check for errors:
package main
import (
"fmt"
"os"
)
func main() {
file, err := os.Open("filename.txt")
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
// Process the file here
}
In the above example, we call the Open
function from the os
package, which opens a file for reading. If an error occurs during the file opening process, the function will return a non-nil error value. We then check if the error is nil and handle it accordingly.
2. Panic and Recover
In some cases, it may be necessary to handle unrecoverable errors that should cause the program to terminate. Go provides the panic
mechanism for this purpose.
The panic
function is used to raise a run-time error that stops the normal execution flow of the program. When panic
is called, the program stops executing and starts unwinding the stack, running deferred function calls along the way.
We can use the recover
function to catch a panic
and resume execution. The recover
function is typically called within a deferred function to handle panics gracefully.
Here's an example of how to use panic
and recover
:
func handlePanic() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}
func doSomething() {
defer handlePanic()
// Some code that may panic
panic("Something went wrong!")
}
In the above example, the doSomething
function panics with a custom message. However, since the panic
is called within a deferred function, the handlePanic
function is called to recover from the panic and continue executing the program. This pattern can be useful for handling unexpected or exceptional errors.
3. Error Handling Patterns
In addition to the techniques mentioned above, there are several error handling patterns commonly used in Go. Let's explore some of these patterns:
3.1 Error Wrapping
Error wrapping is a technique used to add more context to an error by wrapping it with additional information. This can be helpful when propagating errors up the call stack and providing more meaningful error messages.
package main
import (
"errors"
"fmt"
)
func doSomething() error {
err := doSomethingElse()
if err != nil {
return fmt.Errorf("doSomething: %w", err)
}
// Continue with more code
return nil
}
func doSomethingElse() error {
return errors.New("something went wrong")
}
In the above example, the doSomething
function calls doSomethingElse
, which returns an error. Instead of returning the error as is, we use the fmt.Errorf
function to wrap the original error with the additional context of "doSomething"
.
When handling the error, we can use the %w
verb to access the wrapped error:
err := doSomething()
if err != nil {
if errors.Is(err, someError) {
// Handle some error
} else {
// Handle other errors
}
}
3.2 Custom Error Types
Another pattern commonly used in Go is the creation of custom error types. Custom error types allow you to define specific error conditions and handle them accordingly.
type TimeoutError struct {
Timeout time.Duration
}
func (e *TimeoutError) Error() string {
return fmt.Sprintf("Timeout after %v", e.Timeout)
}
func doSomething() error {
return &TimeoutError{Timeout: time.Second * 5}
}
In the above example, we define a custom error type called TimeoutError
with an additional field to represent the timeout duration. By implementing the Error()
method for the TimeoutError
type, we can customize the error message returned when the error is printed.
Conclusion
Handling errors effectively is crucial for writing reliable and robust code in Go. By understanding and applying the techniques and patterns discussed in this blog post, you'll be able to catch and handle errors gracefully, ensuring smooth execution of your Go programs.
Remember, Go's explicit error handling approach helps prevent errors from being ignored, making your code more reliable and maintainable. So, embrace the Go way of error handling and write cleaner and more resilient code!
Thank you for reading. Stay tuned for more Go programming tips and tricks to level up your skills!