Domain Events and Event Sourcing in Golang: A Deep Dive
Welcome to our deep dive into domain events and event sourcing in Golang. In this comprehensive guide, we'll explore the concepts of domain events, their importance, and how to implement event sourcing. By the end, you'll be ready to leverage these techniques in your own projects.
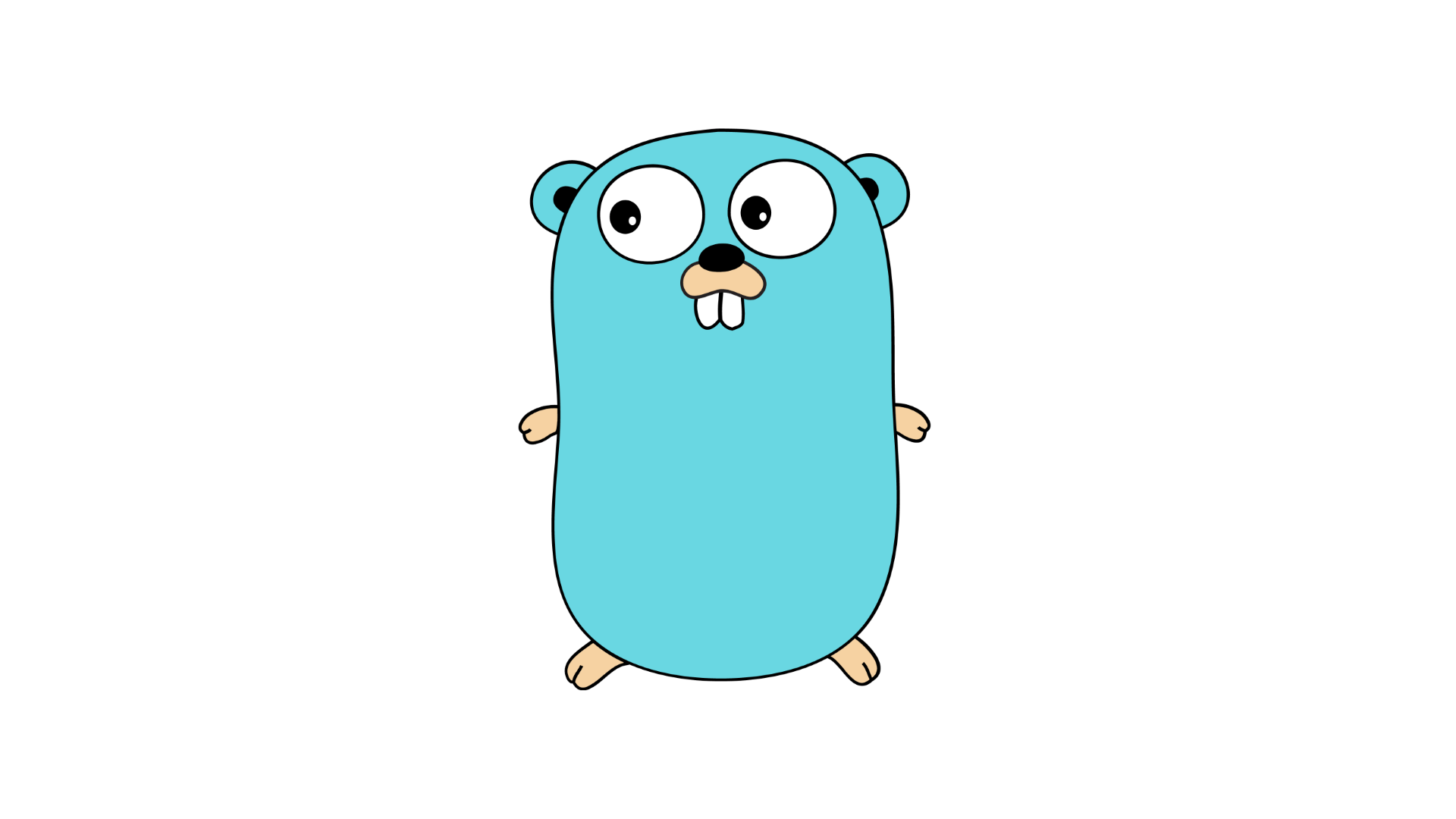
Domain Events and Event Sourcing in Golang: A Deep Dive
Welcome to our deep dive into domain events and event sourcing in Golang. In this comprehensive guide, we'll explore the concepts of domain events, their importance in software development, and how to implement event sourcing in your Golang applications. By the end, you'll have a strong understanding of these powerful techniques and be ready to leverage them in your own projects.
Introduction
In traditional software development, the focus is often on capturing the current state of an application. However, as systems grow in complexity, capturing and understanding the series of events that led to that state becomes increasingly important. This is where domain events and event sourcing come into play.
Domain events are lightweight objects that capture something significant that has happened in your application's domain. They represent facts or state changes that have occurred and are relevant to the business. By capturing these events, you create a record of everything that has happened in your system's history, making it easier to understand and reason about the state of your application.
The Importance of Domain Events
Domain events have several key benefits:
1. Capturing History
By capturing domain events, you create a log of everything that has happened in your system. This historical information can be invaluable for debugging, auditing, and compliance purposes. It allows you to replay events to understand how your system arrived at a certain state, making it easier to diagnose and fix issues.
2. Building Business Intelligence
Domain events capture business-relevant state changes. By analyzing these events, you can gain insights into user behavior, spot patterns, and make data-driven decisions. This can lead to improved business intelligence and better decision-making.
3. Enabling Collaboration
Domain events provide a common language for different parts of your system to communicate. They allow teams to work independently, exchanging events as messages. This promotes loose coupling and enables better collaboration between teams working on different areas of your application.
Implementing Event Sourcing in Golang
Event sourcing is a pattern that builds on top of domain events. It involves storing the state of an application as a series of events, rather than just the current state. This approach has several advantages:
1. Historical State
By storing events instead of just the current state, you can reconstruct past states of your application. This can be useful for creating reports, analyzing historical data, or responding to regulatory requirements.
2. Reproducibility
Since events are immutable, you can always reconstruct the current state of your application by replaying the events from the beginning. This gives you the ability to reproduce any point in time and ensures the integrity of your system.
3. Optimistic Concurrency
In an event sourcing architecture, conflicts are rare. Since events are stored in an append-only manner, multiple writers can safely write events concurrently. In case of conflicts, optimistic concurrency control can be used to resolve them.
Event Sourcing in Golang
Now, let's explore how you can implement event sourcing in Golang:
1. Event Store
The event store is where the domain events are persisted. It is responsible for maintaining the event history and providing methods to retrieve and append events. You can choose from various event store implementations, such as using a relational database, a NoSQL database, or even Apache Kafka.
Example:
type EventStore interface {
AppendEvent(aggregateID string, event Event) error
GetEvents(aggregateID string) ([]Event, error)
}
2. Aggregates
In event sourcing, aggregates are responsible for handling commands and generating events. They encapsulate the business rules, state changes, and event generation. An aggregate should be treated as a transactional boundary to ensure consistency and integrity.
Example:
type Aggregate interface {
Handle(command Command) ([]Event, error)
Apply(event Event)
}
3. Event Bus
The event bus acts as a communication channel between aggregates and other components of your system. It allows you to publish events and subscribe to events, enabling loose coupling and decoupled communication.
Example:
type EventBus interface {
Publish(event Event) error
Subscribe(handler EventHandler) error
}
4. Projections and Read Models
Projections and read models are used to materialize the current state of an aggregate or a specific view of your system. They listen to events and update their own data accordingly. This provides optimized read access and improves performance.
Example:
type UserReadModel struct {
ID string
Name string
Email string
}
// Event Handler for UserCreated event
func (u *UserReadModel) HandleUserCreatedEvent(event UserCreatedEvent) {
u.ID = event.UserID
u.Name = event.Name
u.Email = event.Email
}
Conclusion
Domain events and event sourcing are powerful techniques that can greatly enhance the modularity, scalability, and maintainability of your Golang applications. By capturing relevant business events, leveraging event sourcing, and implementing the necessary components, you can build resilient and flexible systems that provide a complete view of your application's history.
We hope this deep dive into domain events and event sourcing in Golang has provided you with valuable insights and a solid foundation for implementing these concepts in your own projects. Happy coding!