Building Scalable and Maintainable Applications with Domain-Driven Design in Golang
"Learn how to implement Domain-Driven Design (DDD) principles in Golang to create scalable and maintainable applications. Discover the benefits of DDD and key concepts like aggregates, value objects, repositories, and services."
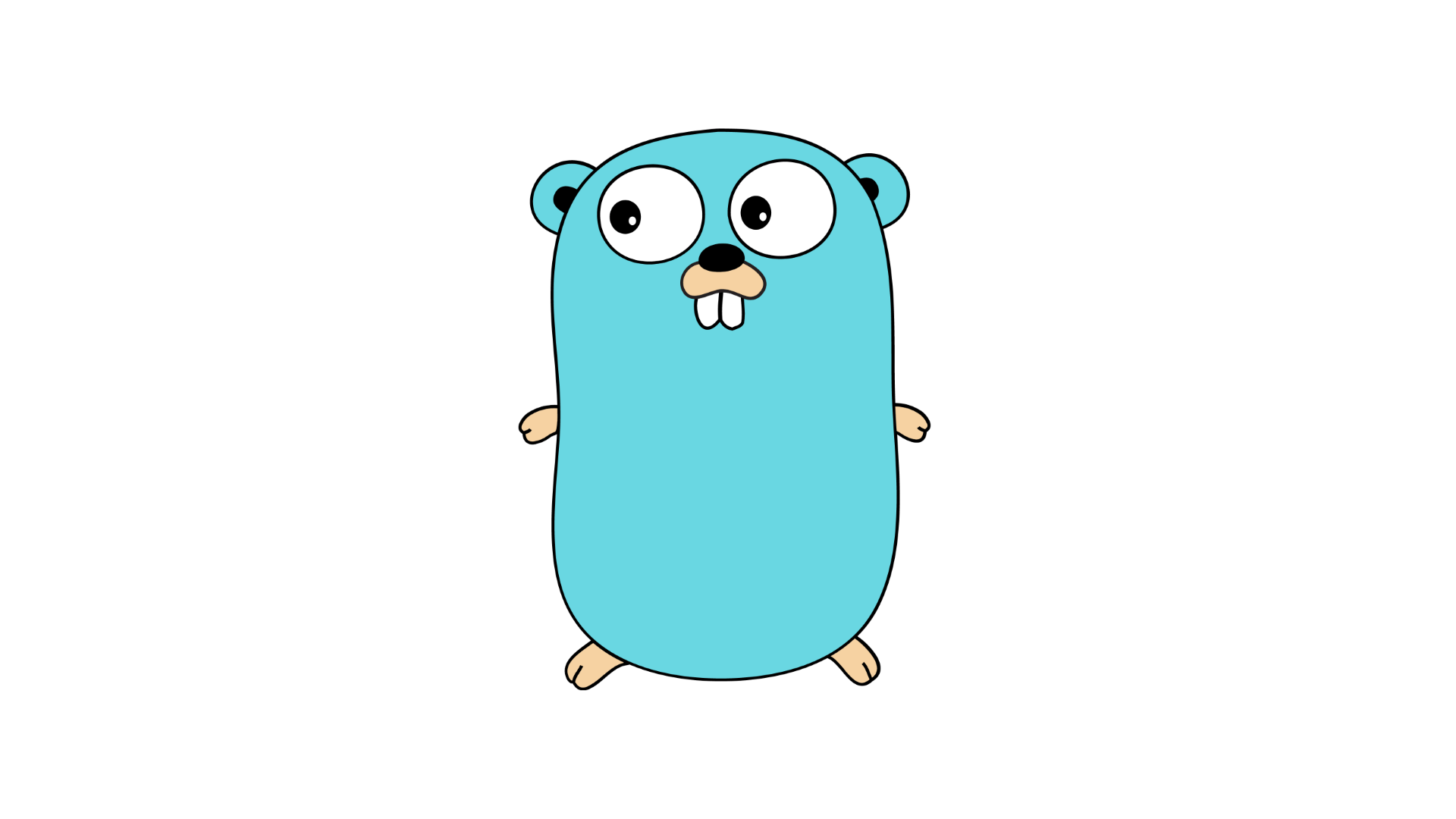
Introduction
Building scalable and maintainable applications is a key goal for every software developer. One way to achieve these objectives is by adopting a software development methodology called Domain-Driven Design (DDD). In this article, we will explore how to implement DDD principles in Golang to create scalable and maintainable applications.
What is Domain-Driven Design?
Domain-Driven Design, or DDD, is an approach to software development that focuses on modeling the core business domain of an application. It was introduced by Eric Evans in his book "Domain-Driven Design: Tackling Complexity in the Heart of Software". The main idea behind DDD is to align the software design with the business domain, helping developers to better understand and solve complex business problems.
Benefits of Domain-Driven Design
Implementing DDD in your application can have several benefits:
- Better communication between business stakeholders and developers.
- Improved problem-solving by leveraging domain knowledge.
- Increased code quality and maintainability.
- Flexibility to handle changing business requirements.
- Scalability and modularity in application design.
Domain-Driven Design in Golang
Golang is a programming language known for its simplicity, efficiency, and strong concurrency features. When it comes to implementing DDD principles in Golang, there are some key concepts and patterns to consider:
1. Aggregates
In DDD, an "aggregate" is a cluster of domain objects that are treated as a single unit. In Golang, an aggregate can be represented by a struct that encapsulates related domain entities and their behavior. For example, a "Order" aggregate may contain a "Customer" entity and multiple "OrderItem" entities.
```go type Order struct { ID string Customer Customer Items []OrderItem } type Customer struct { ID string Name string } type OrderItem struct { ProductID string Quantity int } ```
2. Value Objects
Value objects represent concepts that are immutable and do not have an identity of their own. In Golang, a value object can be implemented as a struct with value semantics. For example, a "Money" value object can encapsulate an amount and a currency.
```go type Money struct { Amount float64 Currency string } ```
3. Repositories
Repositories provide an abstraction for storing and retrieving domain objects. In Golang, repositories can be implemented using interfaces and corresponding repository implementations. For example, a "OrderRepository" interface can define methods for querying and persisting orders.
```go type OrderRepository interface { GetByID(id string) (*Order, error) Save(order *Order) error } type OrderRepositoryImpl struct { // implementation details } ```
4. Services
Services encapsulate behavior that doesn't fit naturally into an entity or value object. In Golang, services can be implemented as standalone functions that operate on domain objects or as methods on the corresponding domain objects.
```go type OrderService struct { OrderRepository OrderRepository } func (s *OrderService) PlaceOrder(order *Order) error { // business logic for placing an order } ```
Structuring Your Golang Application
To effectively apply DDD principles in your Golang application, it is important to have a well-structured project layout:
1. Project Structure
Aim for a modular project structure that reflects the business domain. Each module should have its own directory, containing related models, repositories, services, and interfaces.
``` project/ cmd/ main.go internal/ modules/ order/ models.go repository.go service.go customer/ models.go repository.go pkg/ utils/ errors/ ```
2. Interface Definition
Define interfaces for your modules to promote modularity and testability. Repositories and services should be defined as interfaces so that they can be easily mocked or replaced with alternative implementations.
```go type OrderRepository interface { GetByID(id string) (*Order, error) Save(order *Order) error } type OrderService interface { PlaceOrder(order *Order) error } ```
3. Dependency Injection
Use dependency injection to provide implementations of interfaces to the dependent modules. This allows for loose coupling and easier testing. There are several dependency injection frameworks available for Golang, such as Google's Wire and Uber's Dig.
```go func NewOrderService(orderRepo OrderRepository) OrderService { return &OrderServiceImpl{ OrderRepository: orderRepo, } } ```
Conclusion
Implementing Domain-Driven Design in Golang can significantly improve the scalability and maintainability of your applications. By aligning the software design with the core business domain, you can create more robust and flexible solutions. Remember to focus on aggregates, value objects, repositories, and services, and structure your project in a modular and testable way. Happy coding!