Introduction to Domain-Driven Design with Golang: Best Practices and Benefits
Discover the benefits of implementing Domain-Driven Design (DDD) in your Golang projects. From aggregates to repositories, learn the core concepts and best practices of DDD in this comprehensive guide. Boost maintainability and scalability while fostering collaboration with domain experts.
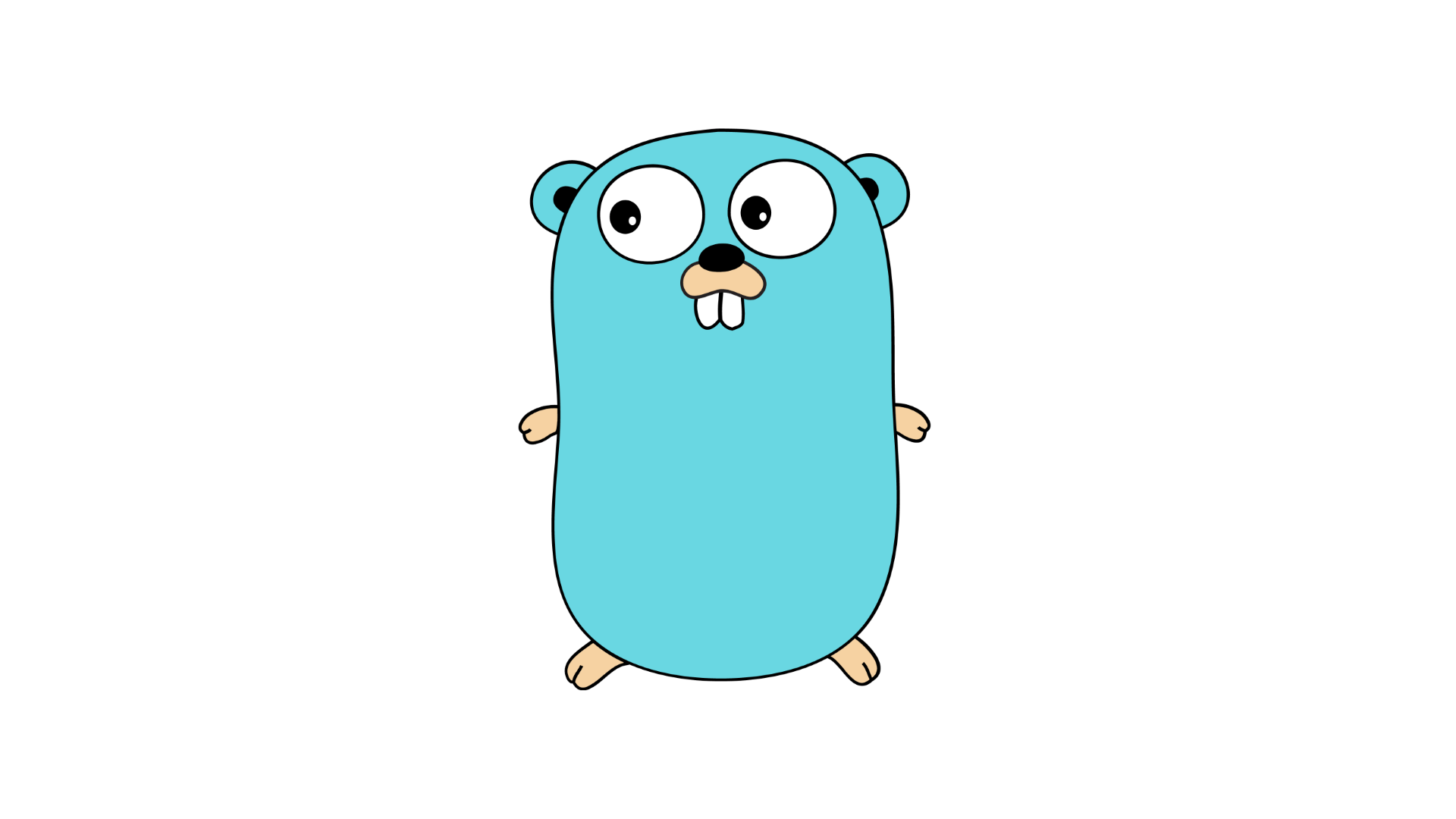
Introduction
Welcome to the world of Domain-Driven Design (DDD) with Golang! In this blog post, we will explore the fundamental concepts of DDD, its best practices, and the benefits of implementing DDD in your Golang projects. Whether you are a beginner or an experienced developer, this guide will provide you with practical insights to help you leverage the power of DDD in your software development journey.
What is Domain-Driven Design?
Domain-Driven Design (DDD) is an architectural approach that focuses on the domain logic and models of a software system. It emphasizes the collaboration between domain experts and developers to create a shared language and understanding of the problem domain. By aligning the software design with the mental models of the domain experts, DDD aims to deliver highly maintainable, flexible, and scalable software solutions.
Core Concepts of DDD
Before diving into the best practices, let's familiarize ourselves with the core concepts of DDD:
1. Aggregates
An aggregate is a cluster of related objects that form a cohesive unit. It represents a transactional consistency boundary within the domain model. Aggregates protect the integrity of their internal state by encapsulating their behavior and ensuring that all invariants are maintained during state changes.
2. Entities
Entities are objects with a unique identity that persists throughout the system's lifecycle. The identity is key to distinguishing entities from one another and ensuring their accurate representation in the domain model. Entities encapsulate their behavior and state, providing operations that adhere to the domain's business rules.
3. Value Objects
Value Objects are objects that represent concepts in the domain but do not have a unique identity. They are immutable and defined solely by their values. Value objects are used for data encapsulation and are frequently utilized as building blocks for entities and aggregates.
4. Repositories
Repositories provide an abstraction layer for accessing and persisting aggregates to storage. They serve as the bridge between the application's business logic and the data access layer. Repositories encapsulate the logic for querying and modifying aggregates, providing a consistent and domain-centric interface to the application.
5. Services
Services represent domain operations or activities that do not naturally belong to a specific entity or value object. They encapsulate business logic and orchestrate the interaction between multiple aggregates or entities. Services promote cohesive and reusable operations within the domain model.
6. Factories
Factories encapsulate the complex creation logic of aggregates, entities, or value objects. They abstract away the construction details, enabling the client code to focus on the essential business logic. Factories ensure the consistency and validity of the created objects based on the domain rules.
Best Practices of Domain-Driven Design in Golang
Implementing DDD in Golang involves following a set of best practices to ensure a clean and domain-centric design. Let's explore these practices:
1. Ubiquitous Language
Establish a shared language and understanding between developers and domain experts. Use a common vocabulary and terminology in the code that reflects the domain's concepts and rules. This language becomes the backbone of the software, aligning the mental models of all stakeholders and reducing communication gaps.
2. Clear Aggregate Boundaries
Define clear aggregate boundaries based on transactional consistency and domain-driven design. Aggregates should be independent and self-contained, encapsulating their invariants. Avoid exposing internal details of an aggregate to other aggregates or external components. Maintain consistency by modifying the aggregates through their defined boundaries.
3. Domain Event-Driven Architecture
Decouple domain operations and enable asynchronous communication by leveraging domain events. Domain events are lightweight messages that represent a significant occurrence within the domain. Events are published by aggregates and consumed by other aggregates or external components. This architecture promotes scalability, extensibility, and loose coupling.
4. Test-Driven Development (TDD)
Adopt Test-Driven Development (TDD) to drive the implementation of the domain models and business logic. Write tests before implementing the functionality, ensuring that the code aligns with the expected behavior. This approach promotes quicker feedback cycles, code comprehension, and maintainability.
5. Scalable Data Access and Persistence
Design a scalable and efficient data access layer by adhering to the principles of DDD. Use repositories to abstract the underlying storage implementation, enabling smooth integration with various database technologies. Implement caching strategies and optimize data retrieval to improve performance and scalability.
Benefits of Domain-Driven Design in Golang
Implementing Domain-Driven Design in your Golang projects offers numerous benefits, including:
1. Increased Maintainability
DDD encourages modular and loosely coupled code, making your codebase easier to maintain. With clear boundaries, encapsulated behavior, and a shared language, developers can easily understand and modify the code without unintended side effects.
2. Scalability and Extensibility
Domain-Driven Design allows for the easy addition of new features and functionalities without impacting existing code. The modular and decoupled nature of DDD promotes scalability, enabling your application to grow and adapt to changing business requirements.
3. Improved Collaboration
By aligning the software design with the domain experts' mental models, DDD fosters collaboration between developers and domain experts. The shared language and understanding facilitate effective communication, leading to better software solutions.
4. Better Code Comprehension
DDD encourages the use of a ubiquitous language and well-defined concepts, making your code more expressive and easier to understand. This improves code comprehension and reduces the time spent on understanding the codebase.
5. Testability and Quality
With DDD's emphasis on TDD, your codebase becomes more testable and less error-prone. The ability to write automated tests for your domain logic ensures a higher level of code quality, leading to reduced bugs and better overall system stability.
Conclusion
Domain-Driven Design offers a powerful approach to develop software solutions that align with the problem domain. By implementing DDD in your Golang projects, you can create maintainable, scalable, and extensible solutions that bridge the gap between developers and domain experts.
Start applying the best practices of DDD in your Golang projects and experience the benefits firsthand. Stay tuned for more articles where we delve deeper into the practical aspects of implementing DDD in Golang. Happy coding!