Debugging and Testing gRPC Services: Best Practices and Tools
Debugging and testing gRPC services is crucial for ensuring reliability. Use logging, enable debug information, leverage distributed tracing, and write comprehensive tests. Explore the best practices and powerful tools available to make your debugging and testing tasks easier and more efficient.
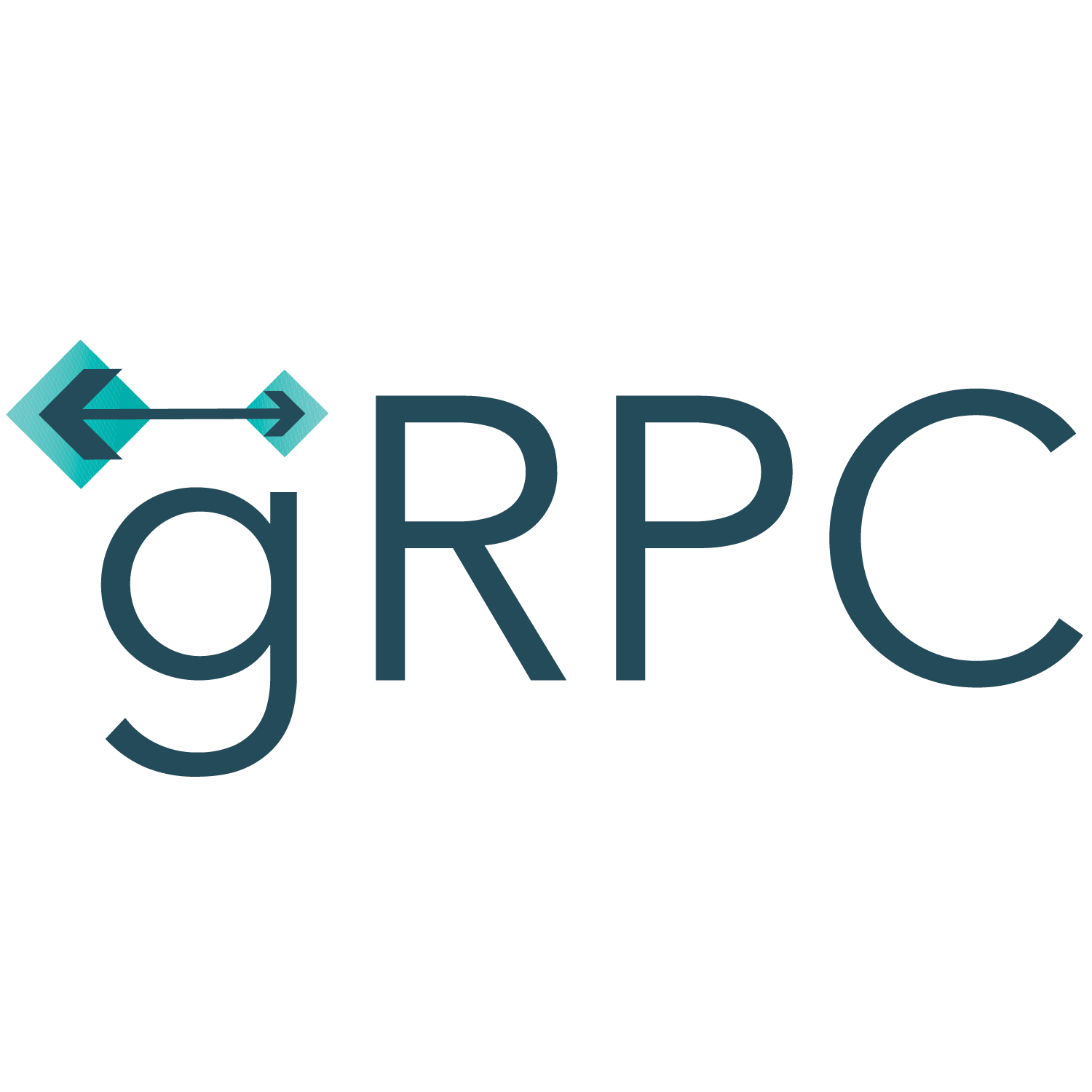
Introduction
Debugging and testing gRPC services is an essential part of ensuring the reliability and quality of your application. As a developer, you need to have a solid understanding of the best practices and tools available to effectively debug and test your gRPC services.
In this blog post, we will explore the best practices for debugging and testing gRPC services, as well as introduce you to some powerful tools that can make your debugging and testing tasks easier and more efficient. Let's dive in!
Debugging gRPC Services
Debugging gRPC services requires a systematic approach and the right tools. Here are some best practices to consider when debugging your gRPC services:
1. Use Logging
Logging is an invaluable tool for debugging any application, and gRPC services are no exception. By incorporating logging statements into your code, you can track the flow of execution and identify any issues or errors that occur along the way. Make sure to log relevant information such as request and response payloads, error messages, and any important variables or states.
Here's an example of how you can use the log
package in Go to log messages:
import "log"
func main() {
// ...
log.Println("Processing request...")
// ...
}
2. Enable Debug Information
Enabling debug information in your gRPC services can provide invaluable insights into the internal workings of your application. By enabling debug logs, you can view detailed information about gRPC calls, including request and response payloads, headers, and any errors that occur during the process.
In Go, you can enable debug logs by setting the environment variable GRPC_GO_LOG_VERBOSITY_LEVEL
to 99
:
$ export GRPC_GO_LOG_VERBOSITY_LEVEL=99
3. Use Distributed Tracing
Distributed tracing allows you to track the flow of requests across multiple services and microservices. By instrumenting your gRPC services with distributed tracing, you can gain valuable insights into the performance and behavior of your application. This can help you identify bottlenecks, latency issues, and potential areas for optimization.
Popular distributed tracing tools for gRPC services include Jaeger and Zipkin. These tools provide a comprehensive view of your application's request flow, allowing you to pinpoint performance issues and identify areas for improvement.
4. Unit Testing
Unit testing is a crucial part of the debugging process. By writing comprehensive unit tests for your gRPC services, you can identify and fix issues before they make their way into production. Make sure to cover all possible edge cases, validate inputs, and simulate different scenarios to ensure the correctness and reliability of your code.
Go provides a built-in testing framework that makes it easy to write and run unit tests for your gRPC services. Here's an example of a unit test written in Go:
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestMyGRPCService_GetData(t *testing.T) {
// ...
// Make gRPC call and assert the response
resp, err := client.GetData(context.Background(), &pb.GetDataRequest{})
assert.NoError(t, err)
assert.NotNil(t, resp)
// ...
}
Testing gRPC Services
Testing gRPC services requires a different approach compared to traditional HTTP-based services. Here are some best practices to consider when testing your gRPC services:
1. Gateway API Testing
The gRPC Gateway provides a way to expose your gRPC services as a RESTful API. This makes it easier to test your gRPC services using familiar HTTP-based testing tools and frameworks, such as cURL or Postman.
By using the gRPC Gateway, you can send HTTP requests to your gRPC services and validate the responses. This allows you to perform thorough API testing, including testing different request methods, headers, and query parameters.
To use the gRPC Gateway, you need to generate the necessary gateway code from your gRPC service definition using the protoc-gen-grpc-gateway
tool. You can then start the gateway server and send HTTP requests to it.
2. Load and Stress Testing
Load and stress testing your gRPC services is essential for evaluating their performance, scalability, and reliability. By simulating high loads and stressing your services, you can identify any performance bottlenecks, capacity limitations, or resource leaks.
Tools like grpcurl
and ghz
can be used to send a large number of concurrent requests to your gRPC services, allowing you to measure the response time, throughput, and error rates.
3. Contract Testing
Contract testing is a technique that ensures the compatibility and consistency between the client and server of a gRPC service. It involves defining a set of contracts or specifications that both the client and server must adhere to.
Tools like protoc-gen-validate
provide a way to define message validation rules in your Protobuf service definition. These rules can be used to validate the incoming requests and responses, ensuring that they meet the expected specifications.
Tools for Debugging and Testing gRPC Services
Here are some powerful tools that can significantly enhance your debugging and testing experience when working with gRPC services:
1. Protoc
protoc
is the protobuf compiler tool that allows you to generate gRPC stub code and message structs from your Protobuf service definition. It is a crucial tool for building gRPC services and clients.
Here's an example of how to use protoc
to generate Go code:
$ protoc --go_out=. --go-grpc_out=. myservice.proto
2. grpcurl
grpcurl
is a command-line tool that allows you to interact with gRPC services using curl-like syntax. It provides an interactive shell for making gRPC calls, inspecting the service schema, and debugging the service.
Here's an example of how to use grpcurl
to make a gRPC call:
$ grpcurl -plaintext -d '{"name":"John"}' localhost:50051 mypackage.MyService/MyMethod
3. ghz
ghz
is a command-line tool that allows you to load test and benchmark your gRPC services. It supports multiple concurrency levels, allows you to specify the payload, and provides various output formats for result analysis.
Here's an example of how to use ghz
to load test a gRPC service:
$ ghz --insecure --proto myservice.proto --call mypackage.MyService/MyMethod -c 10 -n 1000 -data '{"name":"John"}' localhost:50051
4. Jaeger and Zipkin
Jaeger and Zipkin are distributed tracing systems that can be used to trace requests and monitor the performance of your gRPC services. They provide comprehensive dashboards and visualizations that allow you to pinpoint latency issues, bottlenecks, and potential performance optimizations.
Conclusion
Debugging and testing gRPC services is a critical part of the development process. By following the best practices outlined in this blog post and leveraging the right tools, you can ensure the reliability, scalability, and performance of your gRPC services.
Remember to use logging, enable debug information, leverage distributed tracing, and write comprehensive unit tests. Additionally, consider using tools like the gRPC Gateway, load and stress testing tools, and contract testing techniques to enhance your testing process.
With the right mindset and tooling, you'll be well-equipped to tackle any debugging and testing challenges that come your way. Happy debugging and testing!