Command Design Pattern in Golang: Optimizing Domain-Driven Design
The Command Design Pattern in Go optimizes Domain-Driven Design by encapsulating complex operations as commands, promoting modularity and flexibility. Commands provide undo/redo capabilities, separation of concerns, and testability, enhancing the effectiveness of DDD principles.
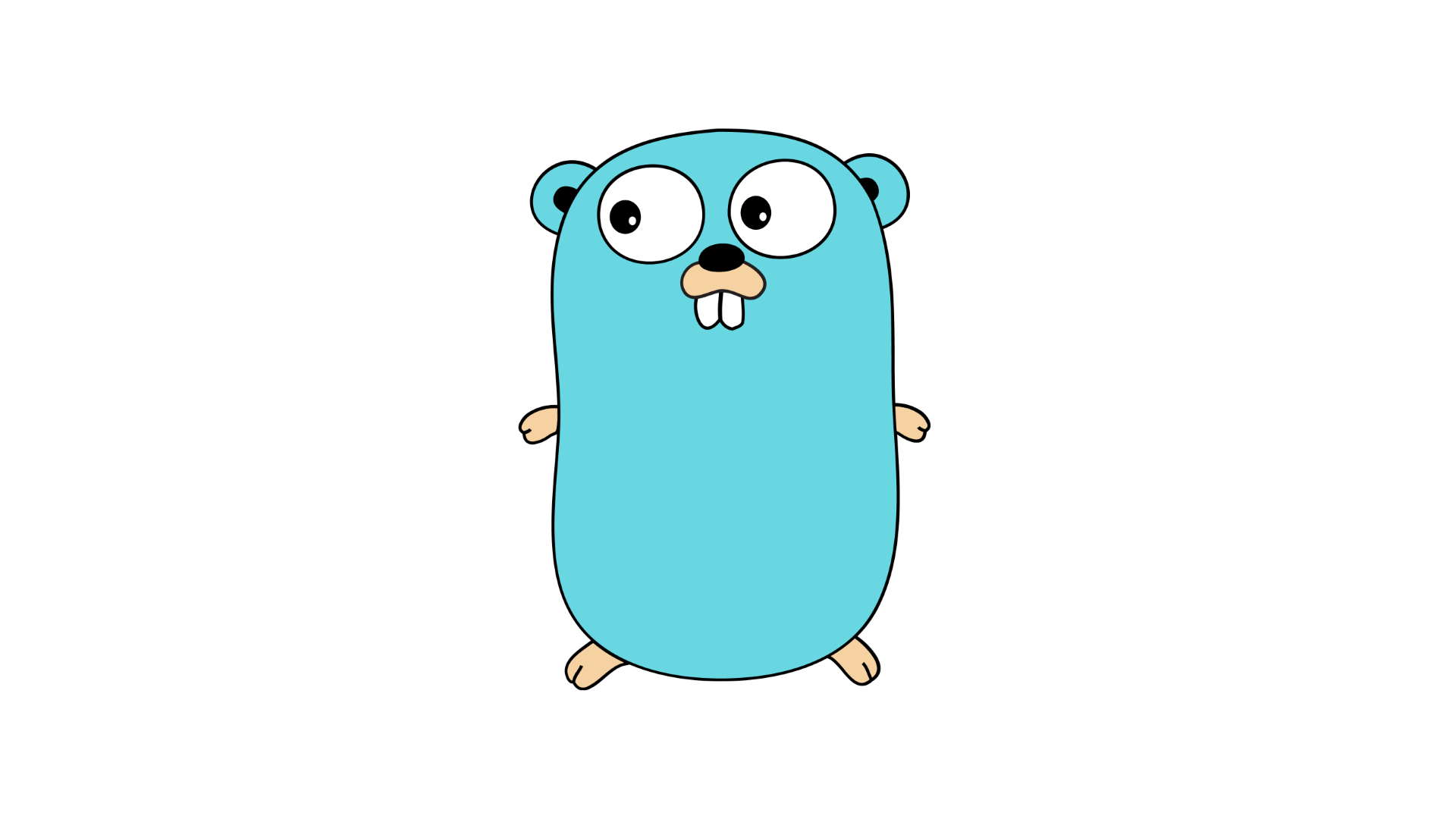
Introduction
The Command Design Pattern is a powerful software design pattern that promotes modularity and extensibility in applications. It is particularly useful in the context of Domain-Driven Design (DDD) where complex business operations are encapsulated as commands. These commands can be invoked, undone, and redone, allowing for flexible and robust behavior in the application.
In this blog post, we will explore the Command Design Pattern in the context of Golang and how it can optimize the implementation of Domain-Driven Design principles. We will discuss the key concepts, benefits, and provide practical examples to help you understand and apply this pattern effectively in your own projects.
The Command Design Pattern
The Command Design Pattern is a behavioral pattern that encapsulates a request as an object, thereby allowing the parameterization of clients with different requests, queue or log requests, and support undoable operations. It decouples the sender of a request from its receiver, providing a way to parameterize clients with different requests, queue or log requests, and support undoable operations.
The key participants in the Command Design Pattern are:
- Command: This is the interface that declares the execution method.
- ConcreteCommand: This is the class that implements the Command interface. It defines the binding between the receiver (the object that performs the operation) and the action to be performed.
- Receiver: This is the class that performs the actual action when the command's execute method is called.
- Invoker: This is the class that asks the command to carry out the request.
- Client: This is the class that creates a concrete command and sets its receiver.
The Command Design Pattern provides several benefits:
- Modularity: Commands encapsulate a specific operation, making it easy to manage and modify.
- Flexibility: Commands can be composed and combined in various ways to achieve complex behavior.
- Undo/Redo: The pattern supports undoable and redoable operations by maintaining a history of commands.
- Separation of Concerns: The pattern decouples the sender of a request from its receiver, promoting loose coupling and modular design.
Implementing the Command Design Pattern in Go
Now let's see how we can implement the Command Design Pattern in Go. We'll start by defining the necessary components:
```go // Command interface type Command interface { Execute() } // ConcreteCommand type CreateOrderCommand struct { orderService OrderService // the receiver order Order // the data required to perform the action } func (c *CreateOrderCommand) Execute() { c.orderService.CreateOrder(c.order) } // Receiver type OrderService struct { // service methods } func (o *OrderService) CreateOrder(order Order) { // implementation logic } // Invoker type OrderInvoker struct { commands []Command } func (oi *OrderInvoker) AddCommand(command Command) { oi.commands = append(oi.commands, command) } func (oi *OrderInvoker) ExecuteCommands() { for _, command := range oi.commands { command.Execute() } } ```
In this example, we define the Command interface and a concrete command, CreateOrderCommand. The CreateOrderCommand struct holds a receiver instance, OrderService, and the data required to perform the action. The Execute method of CreateOrderCommand calls the CreateOrder method on the receiver.
We also define the receiver, OrderService, which contains the actual logic to create an order.
Lastly, we have the invoker, OrderInvoker, which maintains a list of commands and provides methods to add commands and execute them. The ExecuteCommands method iterates over the list of commands and calls the Execute method on each command.
Let's see how we can use these components to create and execute commands:
```go func main() { // create the receiver orderService := &OrderService{} // create the commands createOrderCommand := &CreateOrderCommand{ orderService: orderService, order: Order{ ID: "123", Customer: "John Doe", Items: []string{"Product 1", "Product 2"}, }, } // create the invoker orderInvoker := &OrderInvoker{} // add the command to the invoker orderInvoker.AddCommand(createOrderCommand) // execute the command orderInvoker.ExecuteCommands() } ```
In this example, we create the receiver, OrderService, and the command, CreateOrderCommand, with the necessary data. We then create the invoker, OrderInvoker, and add the command to it using the AddCommand method. Finally, we call the ExecuteCommands method on the invoker to execute the command.
This is a basic example, but you can easily extend it to include more commands and receivers to handle different business operations.
Benefits of Using the Command Design Pattern in Domain-Driven Design
The Command Design Pattern is particularly well-suited for implementing Domain-Driven Design (DDD) principles. Here are some benefits of using this pattern in DDD:
- Separation of Concerns: Commands encapsulate complex business operations, ensuring that the domain logic stays isolated from the application logic.
- Modularity: Each command represents a discrete action in the domain, making it easy to manage and modify.
- Reusability: Commands can be composed and combined to achieve higher-level operations.
- Undo/Redo: The pattern allows for undoable and redoable operations in the domain.
- Testability: Commands can be easily unit tested, helping to ensure the correctness of the business operations.
Conclusion
The Command Design Pattern is a powerful tool for optimizing Domain-Driven Design in Go applications. By encapsulating domain operations as commands, you can achieve modularity, flexibility, and extensibility in your codebase. The separation of concerns provided by this pattern allows for better organization and maintainability of your domain logic.
In this blog post, we explored the key concepts and benefits of the Command Design Pattern. We provided a practical example of how to implement this pattern in Golang, and discussed how it can be effectively used to implement Domain-Driven Design principles.
By leveraging the Command Design Pattern, you can build robust and maintainable applications in Go that align with DDD principles. So go ahead and start applying this pattern in your own projects and see the benefits firsthand!