Using gRPC with GraphQL: Combining the Best of Both Worlds
Learn how to combine the flexibility of GraphQL and the performance of gRPC to create powerful APIs. Discover the benefits and step-by-step guide to setting up a sample project. Harness the best of both worlds in less effort!
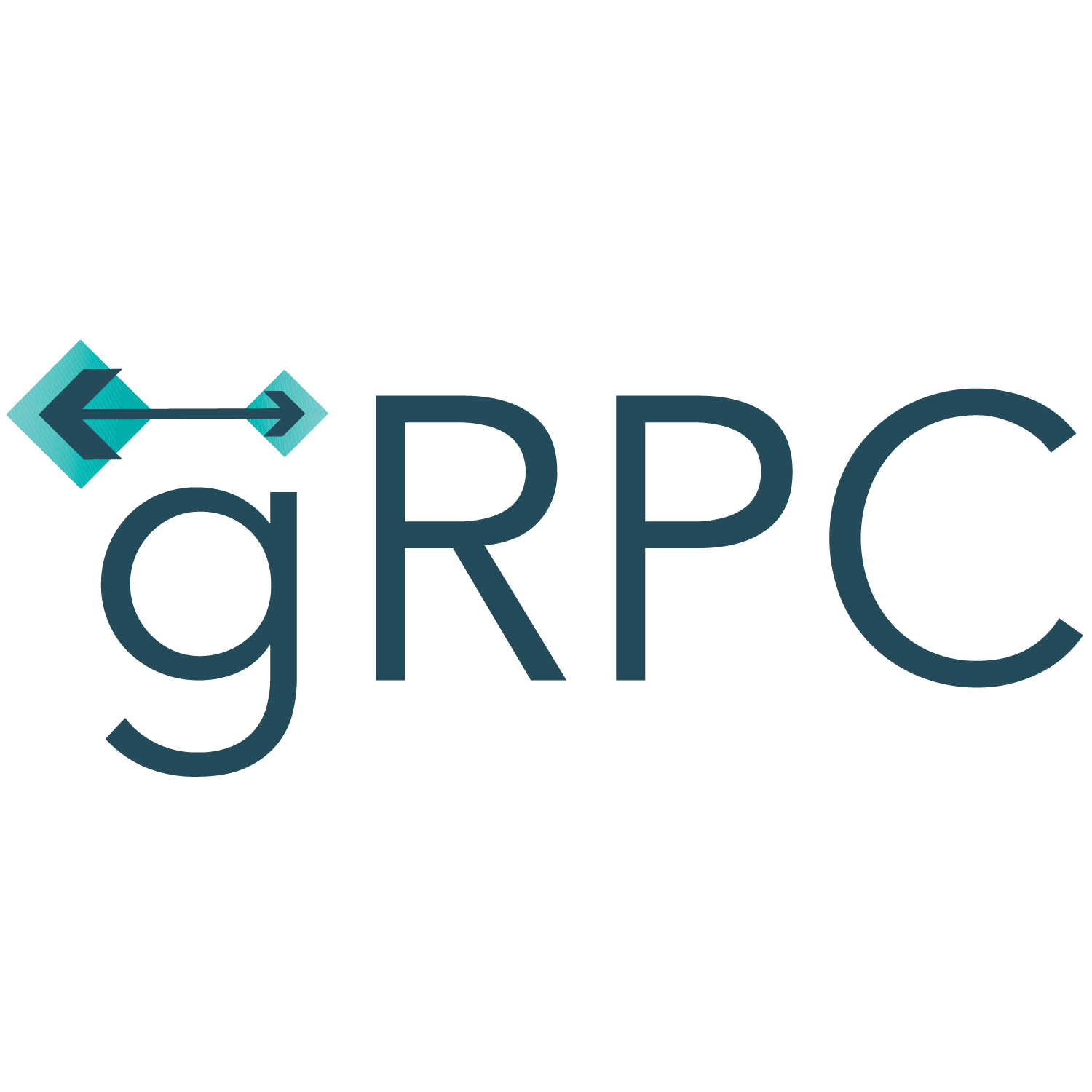
Introduction
As developers, we are always on the lookout for the latest and greatest tools and technologies to enhance our applications. In recent years, both GraphQL and gRPC have gained immense popularity in the world of API development. While GraphQL provides a flexible and efficient way to interact with APIs, gRPC offers a high-performance, language-agnostic remote procedure call framework. But why choose one over the other when we can combine the best of both worlds?
What is GraphQL?
GraphQL is an open-source query language and runtime for APIs. It was developed by Facebook and later open-sourced in 2015. With GraphQL, you can define a schema that describes the capabilities of your API, and clients can query that schema to fetch exactly the data they need, all in a single request. This eliminates the problem of over-fetching or under-fetching data, which is common in traditional RESTful APIs.
What is gRPC?
gRPC is a high-performance, open-source remote procedure call (RPC) framework developed by Google. It allows you to define services using Protocol Buffers (protobufs) and automatically generates client and server code in various programming languages. gRPC uses the HTTP/2 protocol for communication, allowing for efficient and multiplexed requests and responses.
Combining GraphQL and gRPC
While GraphQL and gRPC are powerful tools on their own, combining them can unlock even more possibilities. By using gRPC as the transport layer for GraphQL, we can benefit from the performance and efficiency of gRPC while leveraging the flexibility and declarative nature of GraphQL.
To combine GraphQL and gRPC, we need to define our GraphQL schema using the GraphQL Schema Definition Language (SDL) and generate the necessary code using a code generation tool like the GraphQL Code Generator
. We can then use this generated code to implement the gRPC services and resolvers.
Setting Up a Sample Project
Let's walk through a step-by-step guide on setting up a sample project that combines GraphQL and gRPC:
1. Create a Protobuf Definition
The first step is to define our API using Protocol Buffers. Create a file called api.proto
and define your API as you would in a regular gRPC application. For example:
syntax = "proto3";
package graphql;
message User {
string id = 1;
string name = 2;
}
service UserService {
rpc GetUser (UserRequest) returns (UserResponse) {}
}
message UserRequest {
string id = 1;
}
message UserResponse {
User user = 1;
}
2. Generate the Client and Server Code
Next, we need to generate the client and server code using the protoc
command-line tool. Make sure you have Protocol Buffers installed and run the following command:
$ protoc --proto_path=. --go_out=plugins=grpc:. api.proto
This will generate the necessary Go code for our gRPC server and client.
3. Define the GraphQL Schema
Now, let's define our GraphQL schema using the SDL. Create a file called schema.graphql
and define types and queries that match our gRPC API:
type User {
id: ID!
name: String!
}
type Query {
getUser(id: ID!): User
}
4. Generate the GraphQL Code
Use the GraphQL Code Generator
to generate the necessary code based on our GraphQL schema. Install the GraphQL Code Generator
package globally and run the following command:
$ graphql-codegen init
This will generate a codegen.yml
file. Edit the file and specify the input and output directories:
schema: schema.graphql
generates:
path/to/output/:
- gqlgen
Now, run the following command to generate the GraphQL code:
$ graphql-codegen
5. Implement the gRPC Server and GraphQL Resolvers
Finally, use the generated gRPC server code and GraphQL resolvers to implement the API logic. You can use any programming language supported by gRPC and GraphQL to do this. For example, in Go:
// Implement the gRPC server
type userService struct{}
func (s *userService) GetUser(ctx context.Context, req *pb.UserRequest) (*pb.UserResponse, error) {
// Implement the logic to fetch and return the user
}
// Implement the GraphQL resolver
type Resolver struct{}
func (r *Resolver) GetUser(args struct{ ID string }) (*User, error) {
// Implement the logic to fetch the user using the gRPC client
}
Testing the Combined API
With everything set up, you can now test your combined API. Start the gRPC server and GraphQL server, and use a GraphQL client to send queries to the API. You can test both gRPC and GraphQL queries, using the same API. It's that easy!
Conclusion
By combining the strengths of GraphQL and gRPC, we can build powerful, performant, and flexible APIs. gRPC provides the high-performance transport layer, while GraphQL offers a clean and intuitive way to query and mutate data. By leveraging both technologies, we can create APIs that are efficient, scalable, and easy to work with. So, why choose one when you can have the best of both worlds?
Stay tuned for more tutorials and tips on API development and stay ahead of the curve!