Domain-Driven Design and Test-Driven Development in Golang: A Winning Combination
Learn how combining Domain-Driven Design and Test-Driven Development in Go projects can lead to improved understanding, higher test coverage, and better code design and maintainability.
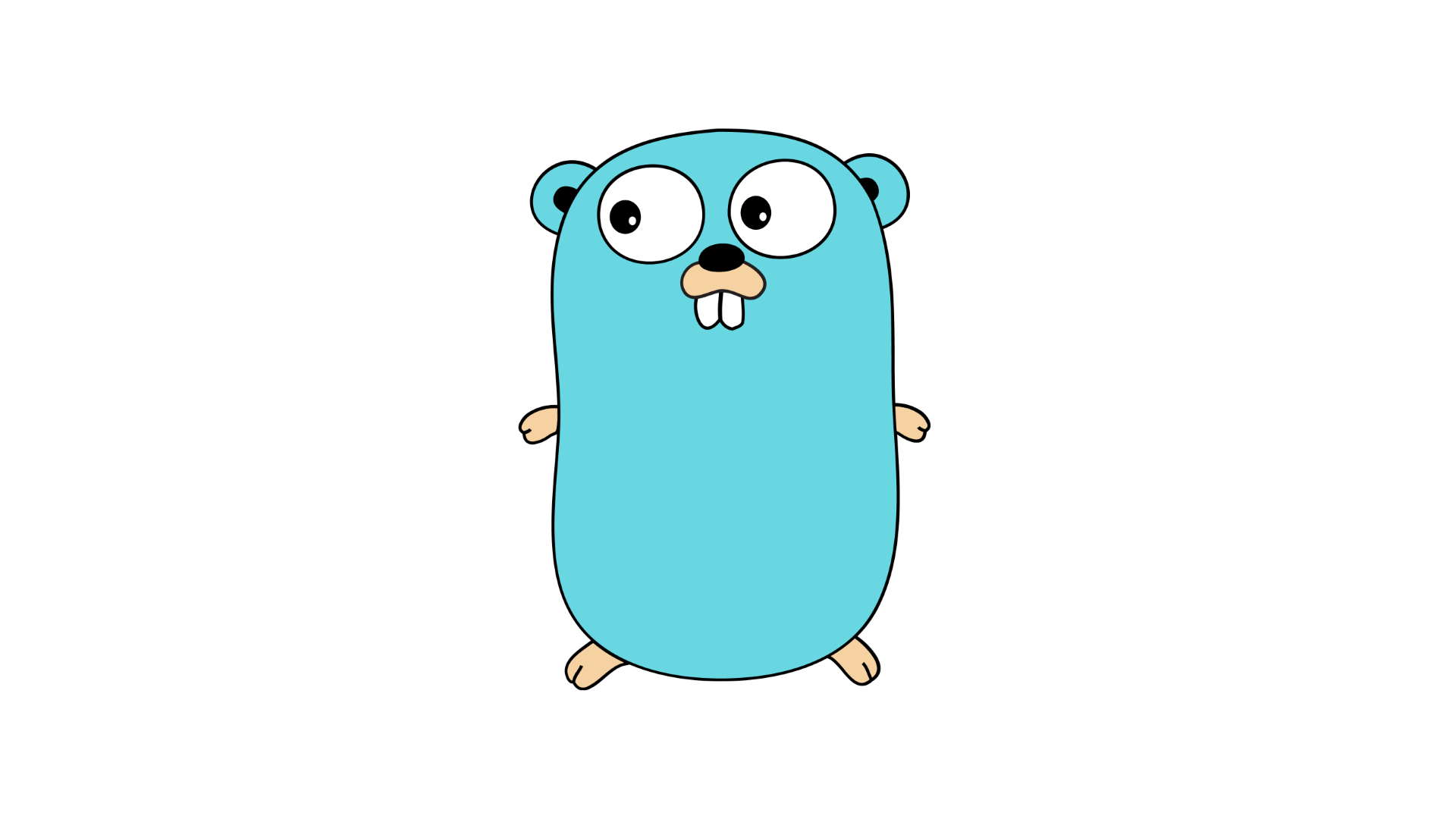
Introduction
Domain-Driven Design (DDD) and Test-Driven Development (TDD) are two powerful methodologies that can significantly improve the software development process. By combining these two approaches, developers can create high-quality codebases that are both maintainable and resilient. In this blog post, we'll explore how DDD and TDD can be effectively applied in Go (Golang) projects, highlighting the benefits of this winning combination.
What is Domain-Driven Design?
Domain-Driven Design is an approach to software development that emphasizes the importance of understanding and modeling the problem domain. It focuses on building software systems that mirror the real-world domain, enabling developers to create solutions that are aligned with the business and its requirements.
The key principles of DDD include:
- Ubiquitous Language: Developers and domain experts should work together to develop a common language that accurately represents the problem domain.
- Model-Driven Design: The domain model plays a central role in DDD. It is a representation of the key concepts, relationships, and business rules of the problem domain.
- Domain Experts: Developers should collaborate with domain experts, who have a deep understanding of the problem domain, to ensure accurate modeling and implementation.
What is Test-Driven Development?
Test-Driven Development is a software development approach in which tests are written before the code. TDD follows a red-green-refactor cycle, where developers first write a failing test, then implement the code to pass the test, and finally refactor the code to improve its design and maintainability.
The primary benefits of TDD include:
- Code Coverage: TDD ensures that all code is thoroughly tested, resulting in high test coverage.
- Regression Prevention: TDD helps identify and fix bugs early, preventing regressions in later stages of development.
- Design Improvements: The iterative nature of TDD encourages developers to write clean, modular, and maintainable code.
The Benefits of Combining DDD and TDD in Golang Projects
Combining Domain-Driven Design and Test-Driven Development can have a profound impact on the quality, maintainability, and scalability of Go projects. Here are some of the key benefits of this winning combination:
1. Improved Understanding of the Problem Domain
By practicing DDD, developers gain a deep understanding of the problem domain, allowing them to create software that accurately captures its complexities and requirements. This understanding is further enhanced by TDD, as developers continually interact with domain concepts while writing tests, reinforcing their knowledge.
2. Enhanced Communication Between Developers and Domain Experts
Domain-Driven Design promotes collaboration between developers and domain experts, fostering a shared understanding of the problem domain. By involving domain experts in the TDD process, developers can gather feedback, validate assumptions, and refine the domain model, leading to more accurate and effective software solutions.
3. Higher Test Coverage and Reduced Bug Risk
Test-Driven Development ensures that every line of code is thoroughly tested, leading to higher test coverage. By writing tests before writing code, developers actively prevent bugs and regressions. This reduces the risk of introducing critical bugs in production and allows for more confident code refactoring and modification.
4. Improved Code Design and Maintainability
TDD encourages developers to write clean, modular, and testable code. By writing tests first, developers are forced to think about the design and structure of their code before implementation. This leads to better code organization, decoupling of dependencies, and improved maintainability.
5. Better Software Architecture and Scalability
DDD promotes the separation of concerns and the creation of modular, loosely coupled components. By applying TDD, developers can ensure that each component is effectively tested and meets the defined requirements. This approach results in a more robust and scalable software architecture, making it easier to introduce new features or modifications to the system.
Practical Tips for Applying DDD and TDD in Golang
Now that we understand the benefits of combining DDD and TDD, let's explore some practical tips for applying these methodologies in Golang projects:
1. Apply Domain-Driven Design Principles
Start by understanding the problem domain and defining the Ubiquitous Language in collaboration with domain experts. Use this knowledge to model the domain using Go structs and define behavior using methods. Organize your codebase into bounded contexts and use repositories to encapsulate data access logic.
2. Write Tests First
Adopt a test-first approach by writing tests before writing the production code. Focus on writing tests that reflect the expected behavior of the system and cover different scenarios. Use testing frameworks like the built-in testing
package in Go to write unit tests, integration tests, and acceptance tests.
3. Apply Test-Driven Development Cycle
Follow the red-green-refactor cycle of TDD. Start with a failing test that represents the desired behavior of the system. Then, implement the minimum amount of code required to pass the test. Finally, refactor the code to improve its design, readability, and maintainability while ensuring that all tests still pass.
4. Continuously Refine the Domain Model
Collaborate with domain experts and iterate on the domain model as the project evolves. Your understanding of the domain will improve over time, and you'll discover new insights and requirements. Refactor the codebase to reflect the refined domain model and ensure that the software remains aligned with the problem domain.
5. Automate Testing and Integration
Invest in automation by setting up a continuous integration (CI) pipeline that runs tests automatically upon code changes. Use tools like GitLab CI, Jenkins, or CircleCI to automate the build, test, and deployment processes. This increases development velocity and ensures that the project remains in a consistent and reliable state.
Conclusion
Domain-Driven Design and Test-Driven Development are two complementary methodologies that can greatly benefit Go projects. By applying DDD, developers can gain a deep understanding of the problem domain and build software that reflects this understanding. Combined with TDD, developers can ensure high code quality, better test coverage, improved maintainability, and an architecture that scales gracefully.
If you're starting a new Go project or working on an existing one, consider adopting the powerful combination of DDD and TDD. You'll find that it leads to better codebases, increased collaboration, and ultimately, more successful software solutions.
Start applying DDD and TDD in your Go projects today, and experience the transformative power of this winning combination!