Building Scalable APIs with gRPC and Protocol Buffers
Learn how to build scalable APIs using gRPC and Protocol Buffers. Discover the advantages of high performance, strong typing, and language-agnostic capabilities.
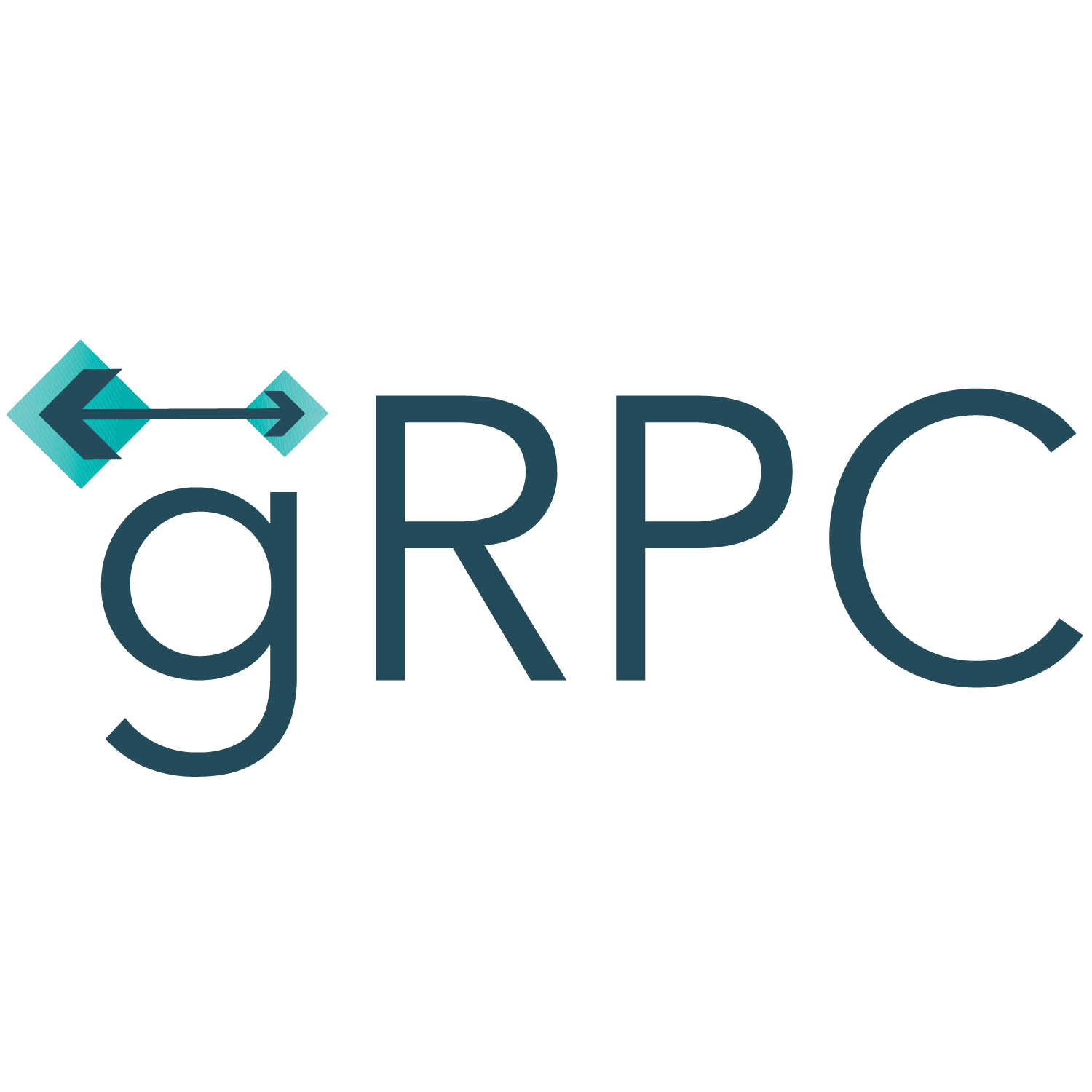
Introduction
Building scalable APIs is crucial for any modern application. As software systems become more distributed and complex, traditional API technologies like REST can start to show their limitations. This is where gRPC and Protocol Buffers come in.
gRPC is a high-performance, open-source framework developed by Google for building APIs. It leverages Protocol Buffers (protobuf) as its interface description language, allowing for efficient serialization and deserialization of structured data. In this blog post, we'll delve into how you can use gRPC and Protocol Buffers to build scalable APIs.
What are gRPC and Protocol Buffers?
Let's start by understanding what gRPC and Protocol Buffers are:
gRPC
gRPC is a modern, high-performance, open-source framework for building remote procedure call (RPC) APIs. It was initially developed by Google and is now a Cloud Native Computing Foundation (CNCF) project supported by a vibrant community.
gRPC allows you to define service contracts using Protocol Buffers and generates client and server code in multiple languages. It uses HTTP/2 as the underlying communication protocol, offering features like bidirectional streaming, flow control, and header compression. These features make gRPC an excellent choice for building low-latency, high-throughput, and efficient APIs.
Protocol Buffers
Protocol Buffers, also known as protobuf, is a language-agnostic binary serialization format developed by Google. It enables efficient and compact serialization of structured data, making it an ideal choice for communication between different systems.
With protobuf, you define your data structures and services in a language-agnostic way using a .proto file. The protobuf compiler then generates code in your desired programming language, allowing you to effortlessly serialize, transmit, and deserialize your structured data. This approach offers strong typing, data validation, and backward compatibility, making protobuf an excellent choice for defining API contracts.
Why Use gRPC and Protocol Buffers for Building Scalable APIs?
Here are some key advantages of using gRPC and Protocol Buffers for building scalable APIs:
High Performance
gRPC leverages the multiplexing and flow control features of HTTP/2, allowing it to handle large numbers of concurrent requests efficiently. The compact binary representation of Protocol Buffers helps reduce network bandwidth and payload size, resulting in faster transmission and lower latencies.
Strong Typing and Validation
Protocol Buffers offer strong typing, allowing you to express complex data structures with ease. The proto compiler can generate code with type checking, ensuring data integrity and eliminating common programming errors. Additionally, protobuf supports data validation rules, enabling you to specify constraints on your data fields.
Backward Compatibility
As your APIs evolve, maintaining compatibility with existing clients becomes crucial. Protocol Buffers handle this gracefully, allowing you to add or remove fields without breaking existing clients. The generated code can handle unknown fields, making it forward and backward compatible.
Language Agnostic
With gRPC and Protocol Buffers, you can define your APIs using a .proto file, which is language-agnostic. This means you can generate client and server code in multiple languages, enabling interoperability between different systems and programming languages.
Building Scalable APIs with gRPC and Protocol Buffers
Now let's dive into the steps involved in building scalable APIs with gRPC and Protocol Buffers:
Step 1: Define Your API
The first step is to define your API using Protocol Buffers. In a .proto file, you specify the data structures and services that make up your API. Here's an example of a simple API definition:
syntax = "proto3";
message Greeting {
string message = 1;
}
service Greeter {
rpc SayHello(Greeting) returns (Greeting);
}
In this example, we define a Greeting
message with a single string field called message
. We also define a Greeter
service with a single RPC method called SayHello
, which takes a Greeting
message as input and returns another Greeting
message.
Step 2: Generate Client and Server Code
Once you have defined your API, you need to generate client and server code in your desired programming language. gRPC provides a protoc compiler that takes your .proto file as input and generates the necessary code. Here's an example command to generate Go code:
protoc --go_out=. --go-grpc_out=. your-api.proto
This command generates Go code that includes the client and server stubs, along with the necessary data structures and methods.
Step 3: Implement the Server
Next, you need to implement the server logic. In Go, you can use the generated code to implement your server. Here's an example implementation for our Greeter
service:
package main
import (
"context"
"log"
"net"
"google.golang.org/grpc"
)
type greeterServer struct{}
func (s *greeterServer) SayHello(ctx context.Context, greeting *pb.Greeting) (*pb.Greeting, error) {
return &pb.Greeting{Message: "Hello, " + greeting.Message}, nil
}
func main() {
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := grpc.NewServer()
pb.RegisterGreeterServer(s, &greeterServer{})
log.Println("Server started on port 50051")
if err := s.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
In this example, we create a new greeterServer
struct that implements the GreeterServer
interface generated by the protoc compiler. We implement the SayHello
method to handle incoming RPC calls. Finally, we start a gRPC server and register our greeterServer
.
Step 4: Implement the Client
Now let's implement a client that can communicate with our server. Here's an example implementation in Go:
package main
import (
"context"
"log"
"google.golang.org/grpc"
)
func main() {
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("failed to connect: %v", err)
}
defer conn.Close()
client := pb.NewGreeterClient(conn)
greeting := &pb.Greeting{Message: "World"}
response, err := client.SayHello(context.Background(), greeting)
if err != nil {
log.Fatalf("failed to call SayHello: %v", err)
}
log.Printf("Response: %s", response.Message)
}
In this example, we dial the gRPC server at localhost:50051
and create a client connection. We then create a new GreeterClient
using the connection and invoke the SayHello
RPC method. Finally, we print the response returned by the server.
Step 5: Run and Test
You can now run your server and client to test the API. Make sure your server is running, and then run your client code. You should see the response printed in the console:
go run server.go
go run client.go
Wrapping Up
gRPC and Protocol Buffers provide a powerful combination for building scalable APIs. By leveraging their high-performance, strong typing, and language-agnostic capabilities, you can create efficient and interoperable API solutions.
In this blog post, we explored the basics of gRPC and Protocol Buffers and went through the steps involved in building scalable APIs. I hope you found this information helpful. Happy coding!