Building RESTful APIs with Golang: A Step-by-Step Tutorial
Learn how to build RESTful APIs with Golang in this step-by-step tutorial. Perfect for beginners, this guide explores the essentials of API development using Golang, providing valuable insights and practical examples. Start building efficient and scalable APIs today!
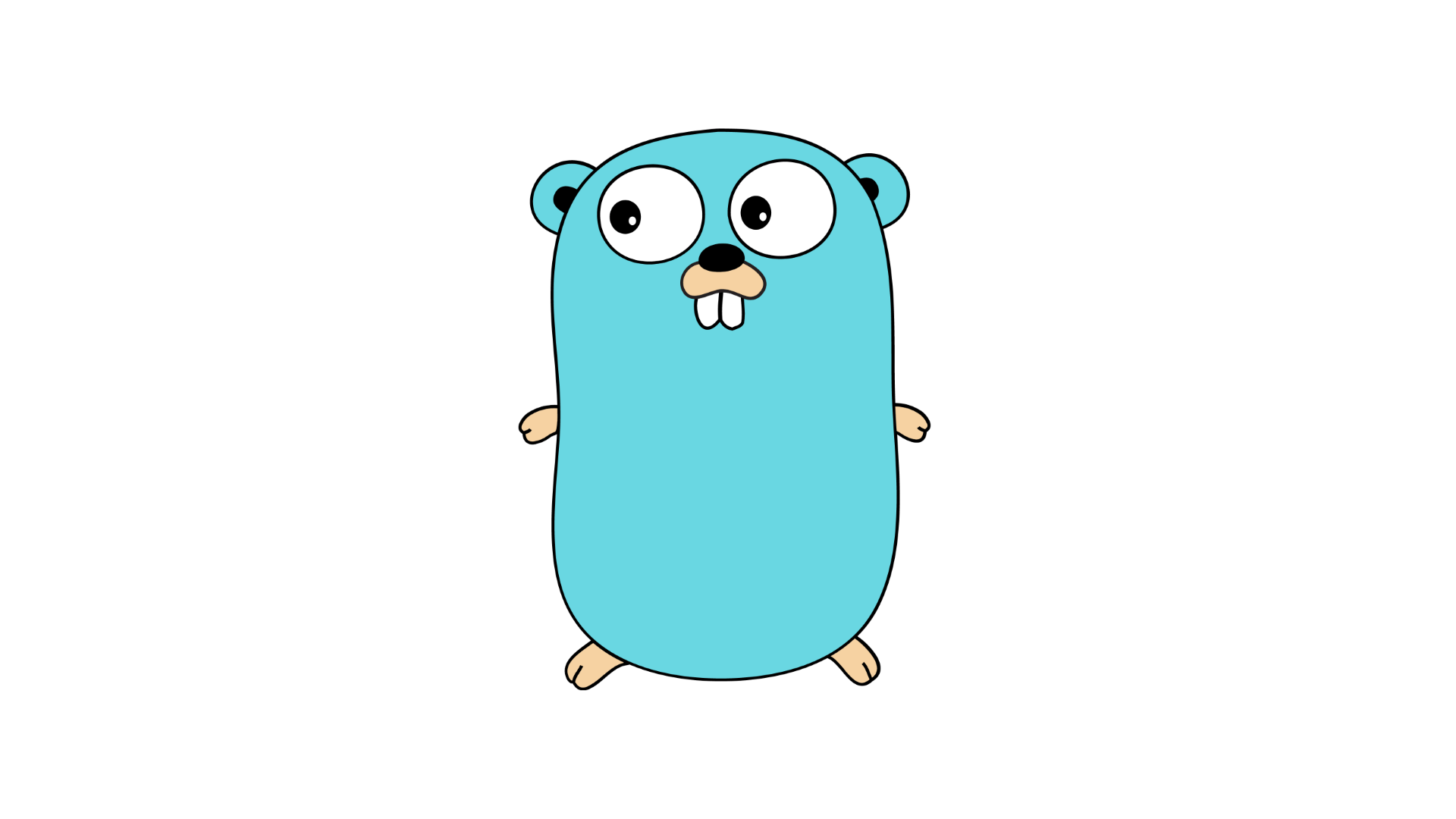
Introduction
Building RESTful APIs with Golang can be a challenging task, especially for beginners. But don't worry, in this step-by-step tutorial we'll guide you through the process and help you understand the intricacies of developing RESTful APIs using Golang.
What are RESTful APIs?
Before we dive into building RESTful APIs with Golang, let's first understand what RESTful APIs are. REST, which stands for Representational State Transfer, is an architectural style that allows systems to communicate over the internet using concise and standardized methods.
RESTful APIs are APIs that adhere to the principles of REST. They enable clients to perform operations on data resources using standard HTTP methods, such as GET, POST, PUT, and DELETE. These APIs can be used to build web services, mobile applications, and various other software applications.
Getting Started
Before we start building RESTful APIs with Golang, make sure you have Golang installed on your machine. You can download and install Golang from the official Golang website. Once Golang is installed, you're ready to get started!
Setting up the Project
The first step in building RESTful APIs with Golang is to set up the project structure. This will help you keep your code organized and maintainable. Let's create a new directory for our project and navigate into it:
$ mkdir my-api
$ cd my-api
Initializing a New Module
Next, we'll initialize a new Go module. This will allow us to manage our project dependencies using Go modules. Run the following command to initialize a new module:
$ go mod init github.com/your-username/my-api
Creating the API Endpoints
Now that our project is set up, it's time to create the API endpoints. API endpoints define the routes and actions that clients can perform on our API. Let's create a file named main.go
and define our API endpoints:
package main
import (
"fmt"
"log"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World!")
}
func main() {
http.HandleFunc("/hello", helloHandler)
log.Fatal(http.ListenAndServe(":8000", nil))
}
In the code above, we define a route "/hello" and a handler function helloHandler
. When a client makes a request to "/hello", the helloHandler
function will be called and it will write "Hello, World!" to the response.
Testing the API
Now that our API endpoints are defined, let's test our API to see if it's working correctly. Open a terminal, navigate to the project directory, and run the following command:
$ go run main.go
This will start the API server on port 8000. Open your web browser and visit http://localhost:8000/hello
. You should see the message "Hello, World!" displayed on the screen.
Building More Complex APIs
So far, we've built a simple API with just one endpoint. But in real-world scenarios, APIs tend to have more complex functionality and multiple endpoints. Here are some tips for building more complex APIs:
1. Structuring Your Code
To keep your code organized and maintainable, it's important to structure your code properly. You can create separate packages for different parts of your API, such as models, handlers, and routes. This will make it easier to understand, test, and maintain your code.
2. Using a Router Package
Golang provides a built-in package called "net/http" for building HTTP servers. However, when it comes to routing, it can be a bit low-level. Consider using a router package, such as Gorilla Mux or Chi, to simplify the routing process and handle more advanced routing features.
3. Implementing Validation and Error Handling
Validating user input and handling errors is crucial in building robust APIs. Use appropriate validation techniques, such as input validation libraries or struct tags, to validate user input. Also, handle errors gracefully by returning meaningful error messages and status codes to clients.
4. Adding Authentication and Authorization
If your API requires authentication and authorization, implement the necessary mechanisms to secure your API. Use industry-standard authentication protocols, such as OAuth 2.0 or JSON Web Tokens (JWT), to authenticate clients. Also, implement role-based access control (RBAC) or other authorization mechanisms to control access to your API endpoints.
Conclusion
Building RESTful APIs with Golang can be a rewarding experience. In this tutorial, we've covered the basics of building a simple API with Golang. We've also discussed some tips for building more complex APIs. Now it's time for you to explore and build your own RESTful APIs using Golang.
Remember to always test your APIs thoroughly and follow best practices for API design and security. Happy coding!