Building Resilient Microservices with gRPC and Circuit Breaker Pattern
Learn how to build resilient microservices with gRPC and the Circuit Breaker pattern. Combine efficient communication with fault tolerance for robust and reliable distributed systems.
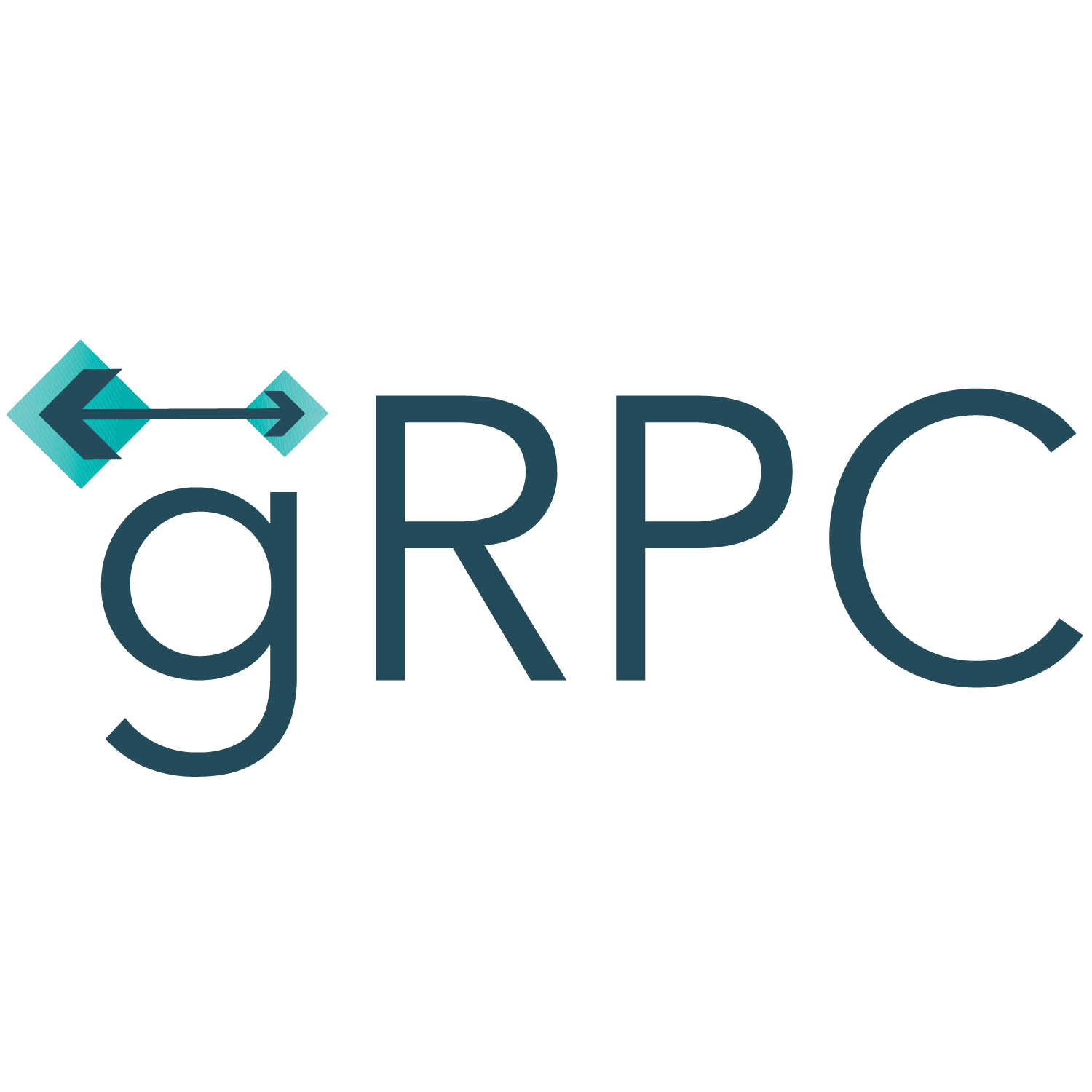
Building Resilient Microservices with gRPC and Circuit Breaker Pattern
Microservices have revolutionized the way we design and develop applications. By breaking down monolithic applications into smaller, independent services, we can achieve scalability, flexibility, and maintainability. However, building microservices comes with its own set of challenges, including network failures and service degradation.
In this blog post, we will explore how to build resilient microservices using gRPC and the Circuit Breaker pattern. By combining the power of gRPC's efficient communication protocol with the fault-tolerant mechanism of the Circuit Breaker pattern, we can create robust and reliable microservices that can handle failures gracefully.
What is gRPC?
gRPC is a high-performance, open-source framework developed by Google for building efficient and scalable microservices. It uses the Protocol Buffers (protobuf) serialization mechanism to define the service contract and message formats, allowing for language-agnostic communication between services.
With gRPC, developers can define service interfaces and data structures using protobuf, and gRPC generates client and server code in various programming languages. This makes it easy to build interoperable microservices in different languages, enabling seamless communication and integration between services.
What is the Circuit Breaker Pattern?
The Circuit Breaker pattern is a proven design pattern for building resilient distributed systems. It acts as a safety net between services, protecting them from cascading failures and preventing system-wide disruptions caused by a failing service.
The Circuit Breaker pattern works by monitoring the calls to a service and maintaining its state. If the service starts to exhibit failures or becomes unresponsive, the Circuit Breaker trips and starts returning predefined fallback responses, instead of making actual calls to the service. This helps prevent overloading the failing service and allows it to recover.
Integrating gRPC and Circuit Breaker
Now that we understand gRPC and the Circuit Breaker pattern, let's see how we can integrate them to build resilient microservices.
Step 1: Define a Protobuf Service
To get started, we need to define our gRPC service using Protobuf. Protobuf allows us to define the service interface and message formats in a language-agnostic way.
```protobuf syntax = "proto3"; package myservice; service MyService { rpc GetData(Request) returns (Response) {} } message Request { // Request fields } message Response { // Response fields } ```
In the above example, we define a simple gRPC service called `MyService` with a single method `GetData`. The `GetData` method takes a `Request` message as input and returns a `Response` message.
Step 2: Implement the gRPC Service
Next, we need to implement the gRPC service in our preferred programming language. gRPC provides client and server libraries for various languages, including Go, Java, Python, and C++.
Here's an example of implementing the `MyService` gRPC service in Go:
```go package main import ( "context" "log" pb "path/to/protobuf" ) type myServiceServer struct{} func (s *myServiceServer) GetData(ctx context.Context, req *pb.Request) (*pb.Response, error) { // Implement the service logic here } func main() { // Initialize gRPC server and register the service } ```
In the above example, we define a Go server that implements the `MyServiceServer` interface generated by gRPC. We implement the `GetData` method to handle incoming requests and return the appropriate response.
Step 3: Implement Circuit Breaker
Now that we have our gRPC service implemented, let's integrate the Circuit Breaker pattern to ensure resilience in the face of failures.
There are several popular Circuit Breaker libraries available for different programming languages, such as Hystrix for Java and Resilience4j for Java and Kotlin. These libraries provide Circuit Breaker implementations with configurable settings like failure thresholds, timeout durations, and fallback responses.
Here's an example of implementing the Circuit Breaker pattern using the Resilience4j library in Java:
```java CircuitBreakerConfig config = CircuitBreakerConfig.custom() .failureRateThreshold(50) .waitDurationInOpenState(Duration.ofMillis(1000)) .permittedNumberOfCallsInHalfOpenState(2) .slidingWindow(10, 100, SlidingWindowType.COUNT_BASED) .build(); CircuitBreaker circuitBreaker = CircuitBreaker.of("myService", config); Supplier decoratedSupplier = CircuitBreaker .decorateSupplier(circuitBreaker, () -> myServiceClient.getData(request)); Response response = Try.ofSupplier(decoratedSupplier) .recover(throwable -> { // Handle fallback response }) .get(); ```
In the above example, we define a `CircuitBreakerConfig` with configurable settings like the failure rate threshold, wait duration, and sliding window. We then create a `CircuitBreaker` instance with the config and use it to decorate the gRPC client call to `GetData`.
If the Circuit Breaker trips due to a high failure rate, the fallback response will be returned instead of making actual calls to the service. This prevents overloading the failing service and allows it to recover.
Summary
By combining the power of gRPC and the Circuit Breaker pattern, we can build resilient microservices that can handle failures gracefully. gRPC provides efficient communication between microservices, while the Circuit Breaker pattern protects services from cascading failures.
Remember to define your gRPC service using Protobuf and implement it in your preferred programming language. Integrate a Circuit Breaker library to add fault tolerance and response fallback capabilities.
Building resilient microservices is crucial for developing robust and reliable distributed systems. By leveraging gRPC and the Circuit Breaker pattern, you can ensure the resiliency of your microservices architecture and deliver a seamless experience to your users.
Happy coding!