Redis Pub/Sub: Building Real-Time Communication with Redis
Redis Pub/Sub enables real-time communication in web applications without the complexity of managing low-level protocols. Learn how to build real-time communication using Redis Pub/Sub and take your applications to the next level.
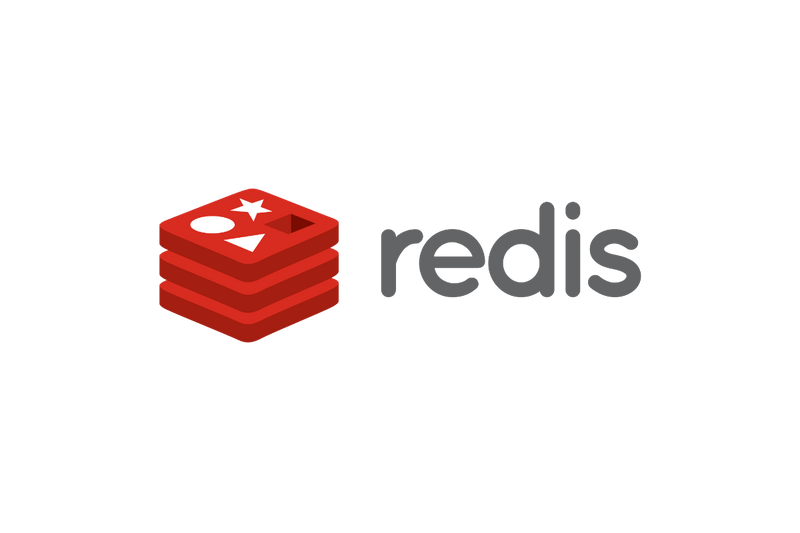
Introduction
Real-time communication is a fundamental requirement for modern web applications. Whether it's chat applications, real-time gaming, or collaboration tools, being able to push updates to clients in real-time is crucial for a rich user experience.
Traditionally, implementing real-time communication involves complex processes like setting up and managing web sockets or implementing long-polling techniques. However, with Redis Pub/Sub, you can easily build real-time communication in your applications without the hassle of managing low-level protocols.
In this article, we'll dive into the world of Redis Pub/Sub and explore how you can leverage its powerful capabilities to build real-time communication in your applications. Let's get started!
What is Redis Pub/Sub?
Redis Pub/Sub is a messaging system that allows applications to send and receive messages in a pub/sub (publish/subscribe) pattern. In this pattern, publishers send messages to specific channels, and subscribers listen to those channels for any updates.
The key advantage of using Redis Pub/Sub is its simplicity and scalability. Redis handles all the heavy lifting involved in managing connections, message queues, and delivery. This allows developers to focus on building and deploying real-time applications without worrying about the complexities of managing message queues.
Setting up Redis
Before we dive into building real-time communication with Redis Pub/Sub, let's set up Redis on our local development environment. Follow these steps:
- Download and install Redis from the official Redis website (https://redis.io/download).
- Start the Redis server in your terminal or command prompt using the following command:
redis-server
Redis will start running on the default port 6379 on your local machine.
Publishing and Subscribing to Channels
Now that we have Redis up and running, let's explore how to use the Pub/Sub feature. We'll start by setting up a publisher that sends messages to a channel, and then we'll create a subscriber that listens to that channel for updates.
Setting up the Publisher
To set up a publisher, open another terminal or command prompt and start a Redis client using the following command:
redis-cli
Once the Redis client is running, use the following command to publish a message to a specific channel:
PUBLISH channel_name "Your message goes here"
Replace channel_name
with the desired name of your channel, and replace "Your message goes here"
with the message you want to send. For example:
PUBLISH notifications "New message received"
After executing the command, the message will be published to the specified channel.
Setting up the Subscriber
Now that we have a publisher set up, let's create a subscriber that listens to the same channel for updates. In a new terminal or command prompt, start another Redis client using the following command:
redis-cli
Once the Redis client is running, use the following command to subscribe to the channel:
SUBSCRIBE channel_name
Replace channel_name
with the name of the channel you want to subscribe to. For example, if you want to subscribe to the "notifications" channel:
SUBSCRIBE notifications
After executing the command, the subscriber will start listening to the specified channel for new messages. Any messages published to the channel will be received by the subscriber in real-time.
Building Real-Time Communication
Now that we understand the basics of Redis Pub/Sub, let's explore how we can use it to build real-time communication in our applications. Here's an example:
```python import redis # Connect to Redis server r = redis.Redis() # Publish a message to a channel r.publish("chatroom", "Hello everyone!") # Create a subscriber p = r.pubsub() p.subscribe("chatroom") # Listen for new messages for message in p.listen(): print(message["data"]) ```
In this example, we first connect to the Redis server using the redis.Redis()
method. Next, we publish a message to the "chatroom" channel using the r.publish()
method. Finally, we create a subscriber using the r.pubsub()
method and subscribe to the "chatroom" channel using the p.subscribe()
method. We then listen for new messages using a loop and print them to the console.
This simple example demonstrates how easily we can implement real-time communication using Redis Pub/Sub. You can extend this functionality to build chat applications, real-time dashboards, and more.
Scaling and Performance
Redis Pub/Sub is designed to be highly scalable and performant. However, as with any distributed system, there are considerations to keep in mind when scaling your applications:
- Ensure you have enough Redis server instances to handle the message load. This will prevent bottlenecks and ensure a smooth experience for your users.
- Consider using a message broker like Redis Streams or Apache Kafka for high-volume applications. These systems provide additional features for managing large volumes of messages.
- Optimize your code and architecture to minimize unnecessary message processing and network overhead.
Conclusion
Redis Pub/Sub is a powerful tool for building real-time communication in your applications. By leveraging its simple yet scalable pub/sub pattern, you can easily push updates and messages to clients in real-time. Whether you're building chat applications, real-time dashboards, or collaborative tools, Redis Pub/Sub is an excellent choice for implementing real-time communication. Give it a try in your next project, and take your applications to the next level!