Building Real-time Chat Applications with Golang: Technologies and Frameworks
Explore the tools and frameworks available in the Go ecosystem for building real-time chat applications. Learn about websockets, real-time messaging platforms, and web frameworks like Gin and Echo. Build robust and scalable chat applications with Go.
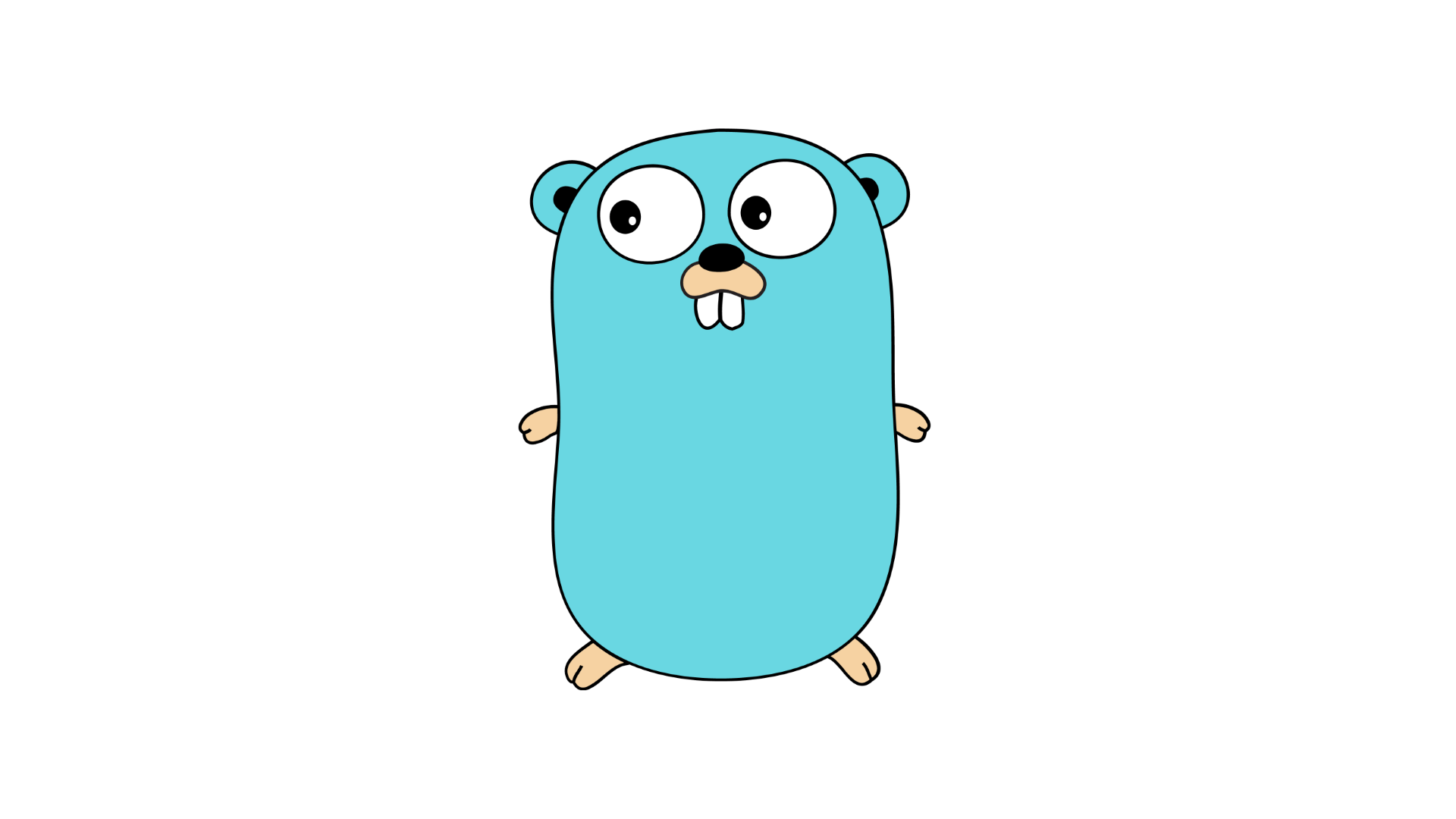
Introduction
Building a real-time chat application can be a challenging but rewarding task. With the rise in demand for instant communication, chat applications have become increasingly popular. In this blog post, we will explore the technologies and frameworks available in the Go (Golang) ecosystem for building real-time chat applications. Whether you are a beginner or an experienced developer, this guide will provide valuable insights into the tools and techniques you can use to create robust and scalable chat applications. Let's dive in!
1. Websockets
Websockets are a key technology for building real-time chat applications. They provide a bidirectional communication channel between the client and the server, enabling instant and continuous data transmission. Go has excellent support for websockets, making it an ideal choice for building real-time chat applications.
One of the most popular Go packages for websockets is the gorilla/websocket
package. It provides a high-level API for working with websockets and offers features like concurrency and message compression. Here is an example of how you can use the gorilla/websocket
package to handle websockets in your Go chat application:
package main
import (
"fmt"
"log"
"net/http"
"github.com/gorilla/websocket"
)
var upgrader = websocket.Upgrader{
ReadBufferSize: 1024,
WriteBufferSize: 1024,
}
func main() {
http.HandleFunc("/websocket", handleWebsocket)
log.Fatal(http.ListenAndServe(":8080", nil))
}
func handleWebsocket(w http.ResponseWriter, r *http.Request) {
conn, err := upgrader.Upgrade(w, r, nil)
if err != nil {
log.Println(err)
return
}
for {
// Read message from the client
_, message, err := conn.ReadMessage()
if err != nil {
log.Println(err)
return
}
// Print the message
fmt.Println(string(message))
// Write message back to the client
err = conn.WriteMessage(websocket.TextMessage, message)
if err != nil {
log.Println(err)
return
}
}
}
2. Real-time Messaging Platforms
Building a chat application from scratch can be time-consuming and complex. Luckily, there are several real-time messaging platforms and frameworks available that provide a ready-to-use infrastructure for building chat applications. These platforms offer features like message synchronization, presence detection, and notification systems, making it easier to create scalable and reliable chat applications.
One popular real-time messaging platform is Pusher. Pusher offers a simple and scalable API for building real-time applications. It provides libraries for various programming languages, including Go, making it easy to integrate with your Go chat application. Here is an example of how you can use Pusher in your Go chat application:
package main
import (
"fmt"
"log"
"net/http"
"github.com/pusher/pusher-http-go"
)
var client = pusher.Client{
AppID: "your-app-id",
Key: "your-app-key",
Secret: "your-app-secret",
Cluster: "your-app-cluster",
}
func main() {
http.HandleFunc("/", handleIndex)
http.HandleFunc("/message", handleMessage)
log.Fatal(http.ListenAndServe(":8080", nil))
}
func handleIndex(w http.ResponseWriter, r *http.Request) {
http.ServeFile(w, r, "index.html")
}
func handleMessage(w http.ResponseWriter, r *http.Request) {
message := r.FormValue("message")
err := client.Trigger("chat", "message", message)
if err != nil {
log.Println("Failed to send message:", err)
}
}
3. Web Frameworks
Web frameworks can greatly simplify the development process when building real-time chat applications. They provide a solid foundation for handling routing, request handling, and other common web development tasks. Here are two popular web frameworks in the Go ecosystem that you can use for building chat applications:
3.1 Gin
Gin is a lightweight and fast web framework for Go. It provides a minimalistic yet powerful API for building web applications. Gin supports features like routing, middleware, and JSON serialization, making it an excellent choice for building real-time chat applications. Here is an example of how you can use Gin to build a simple chat application:
package main
import (
"github.com/gin-gonic/gin"
)
func main() {
router := gin.Default()
router.GET("/", func(c *gin.Context) {
c.HTML(http.StatusOK, "index.html", nil)
})
router.GET("/ws", func(c *gin.Context) {
handleWebsocket(c.Writer, c.Request)
})
router.Run(":8080")
}
3.2 Echo
Echo is another popular web framework for Go that is known for its simplicity and ease of use. It provides a high-performance API for building web applications and has built-in support for websockets. Echo is a great choice if you want a lightweight and fast web framework for your chat application. Here is an example of how you can use Echo to build a chat application:
package main
import (
"github.com/labstack/echo/v4"
"github.com/labstack/echo/v4/middleware"
)
func main() {
e := echo.New()
e.Static("/", "static")
e.GET("/ws", handleWebsocket)
e.Use(middleware.Logger())
e.Use(middleware.Recover())
e.Start(":8080")
}
4. Deploying and Scaling Real-time Chat Applications
Deploying and scaling real-time chat applications is crucial for ensuring optimal performance and reliability. There are several strategies you can employ to deploy and scale your Go chat application:
- Containerization: Use containerization technologies like Docker to package your chat application along with its dependencies into a lightweight and portable container. Containers provide isolation and make it easy to deploy and scale your application.
- Load balancing: Implement a load balancing mechanism to distribute incoming connections across multiple instances of your chat application. This helps distribute the load and improves performance and availability.
- Elastic scaling: Use auto-scaling capabilities provided by cloud platforms like AWS or Kubernetes to automatically adjust the number of instances based on the load. This ensures that your chat application can handle spikes in traffic.
Conclusion
Building real-time chat applications with Go can be an exciting and fulfilling experience. In this blog post, we explored the technologies and frameworks available in the Go ecosystem for building real-time chat applications. We covered websockets, real-time messaging platforms like Pusher, and web frameworks like Gin and Echo that can greatly simplify the development process.
By leveraging these tools and techniques, you can create robust and scalable chat applications that provide a seamless and interactive user experience. So go ahead and start building your own real-time chat application with Go!