Building Command Line Interfaces (CLI) with Golang: A Step-by-Step Tutorial
Learn how to build powerful and user-friendly Command Line Interfaces (CLI) with Golang in this step-by-step tutorial. Perfect for beginners, this tutorial will guide you through the process of creating CLI applications using the Go programming language.
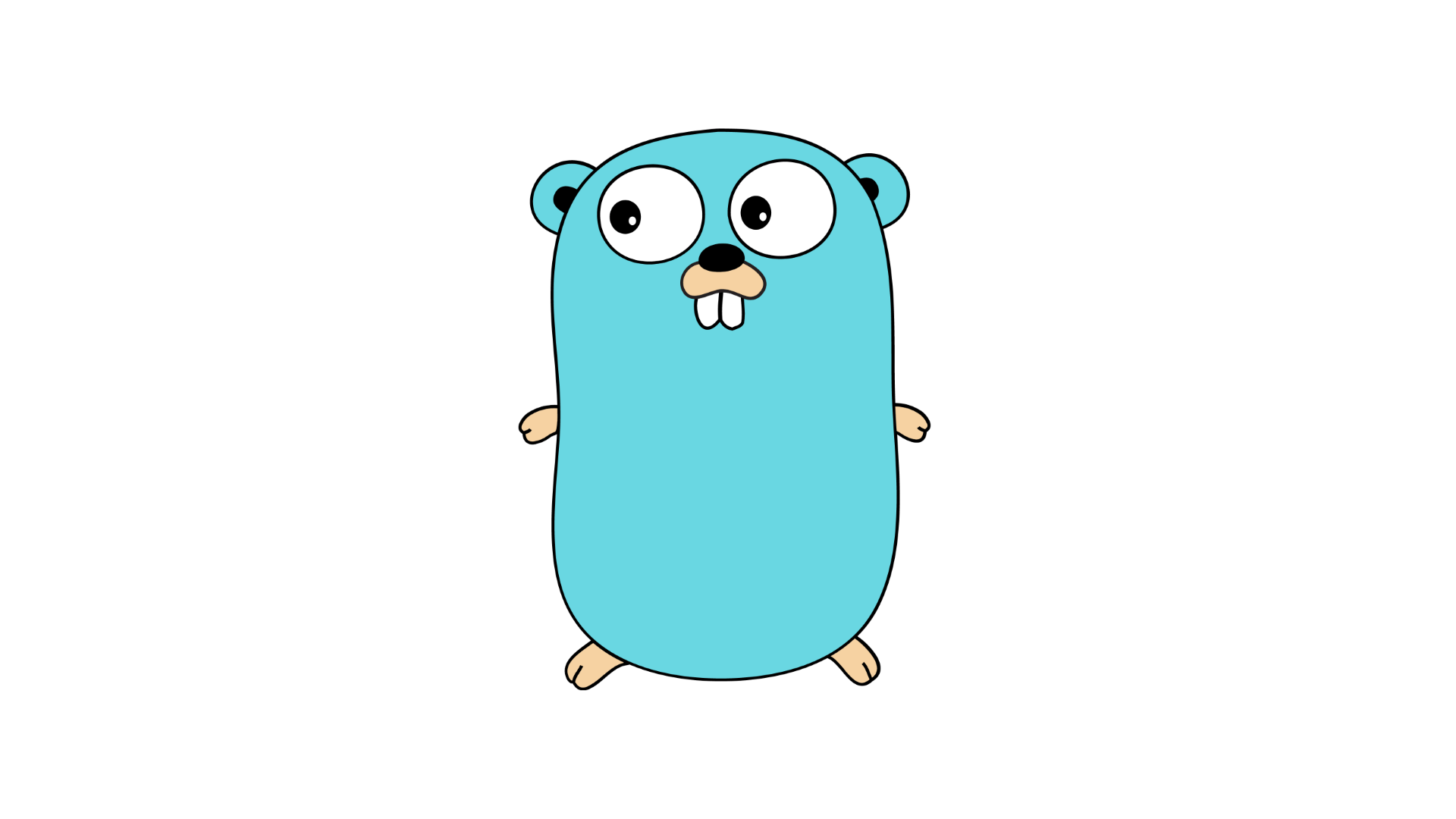
Building Command Line Interfaces (CLI) with Golang: A Step-by-Step Tutorial
Welcome to our step-by-step tutorial on building command line interfaces (CLI) with Golang! In this comprehensive guide, we'll walk you through the process of creating powerful and user-friendly CLI applications using the Go programming language. Whether you're a beginner or an experienced developer looking to expand your skill set, this tutorial will provide you with the knowledge and tools you need to get started with CLI development in Go.
Introduction
Before we dive into the tutorial, let's take a moment to understand what a command line interface (CLI) is and why it's important. A CLI is a text-based user interface that allows users to interact with a computer program through predefined commands. It's a powerful tool that provides a lightweight and efficient way to control and automate various tasks on a computer.
Go, also known as Golang, is a modern programming language developed by Google. It's built with simplicity, performance, and efficiency in mind, making it an excellent choice for building CLI applications. In this tutorial, we'll leverage Go's rich standard library, as well as some popular third-party packages, to create our CLI.
Setting Up the Environment
Before we begin coding our CLI application, we need to set up our development environment. Ensure that you have Go installed on your machine by following the official installation guide for your specific operating system.
Installing Go
Let's start by installing Go on your machine. Follow the steps below:
- Visit the official Go downloads page.
- Choose the package suitable for your operating system and architecture.
- Download the installer and run it.
- Follow the on-screen instructions to complete the installation.
- Verify that Go is successfully installed by opening a terminal and typing
go version
. You should see the installed version of Go displayed.
Creating the CLI Project
Now that we have Go installed, let's create our CLI project. Follow the steps below:
- Create a new directory for your project. You can choose any name for the directory. For example,
my-cli-app
. - Navigate to the newly created directory using the terminal or command prompt.
- Initialize a new Go module by running the command
go mod init github.com/your-username/my-cli-app
. This creates a newgo.mod
file that tracks the dependencies of our project.
Building Your First CLI Command
Now that our project is set up, let's start building our first CLI command. In this example, we'll create a simple command that prints "Hello, World!" when executed.
- Create a new Go file in your project directory and name it
main.go
. - Open the
main.go
file in a text editor or integrated development environment (IDE). - Add the following code to the
main.go
file:
```go package main import "fmt" func main() { fmt.Println("Hello, World!") } ```
Save the file and return to the terminal or command prompt.
Compiling and Running the CLI Command
With our CLI command code in place, let's compile and run it. Follow the steps below:
- Ensure you're in the project directory in the terminal or command prompt.
- Compile the Go code by running the command
go build -o my-cli-app
. This creates an executable file namedmy-cli-app
. - Run the compiled CLI command by executing
./my-cli-app
on Unix-based systems ormy-cli-app.exe
on Windows. You should see "Hello, World!" printed in the console output.
Next Steps
Congratulations! You've successfully built your first CLI command in Go. This is just the beginning of your CLI development journey. Here are some ideas to explore and improve your CLI application:
- Add additional commands and functionalities to your CLI.
- Implement flags and arguments for command-line options and parameters.
- Explore popular CLI frameworks and libraries in the Go ecosystem.
- Learn about input validation and error handling in CLI applications.
Remember to consult the official Go documentation and explore online resources for further information on CLI development in Go.