Best Practices for Error Handling in Golang: Principles and Patterns
Learn the best practices and patterns for error handling in Golang. Understand the principles, patterns, and techniques for effective error handling in Go code, and discover how to handle errors in concurrent applications.
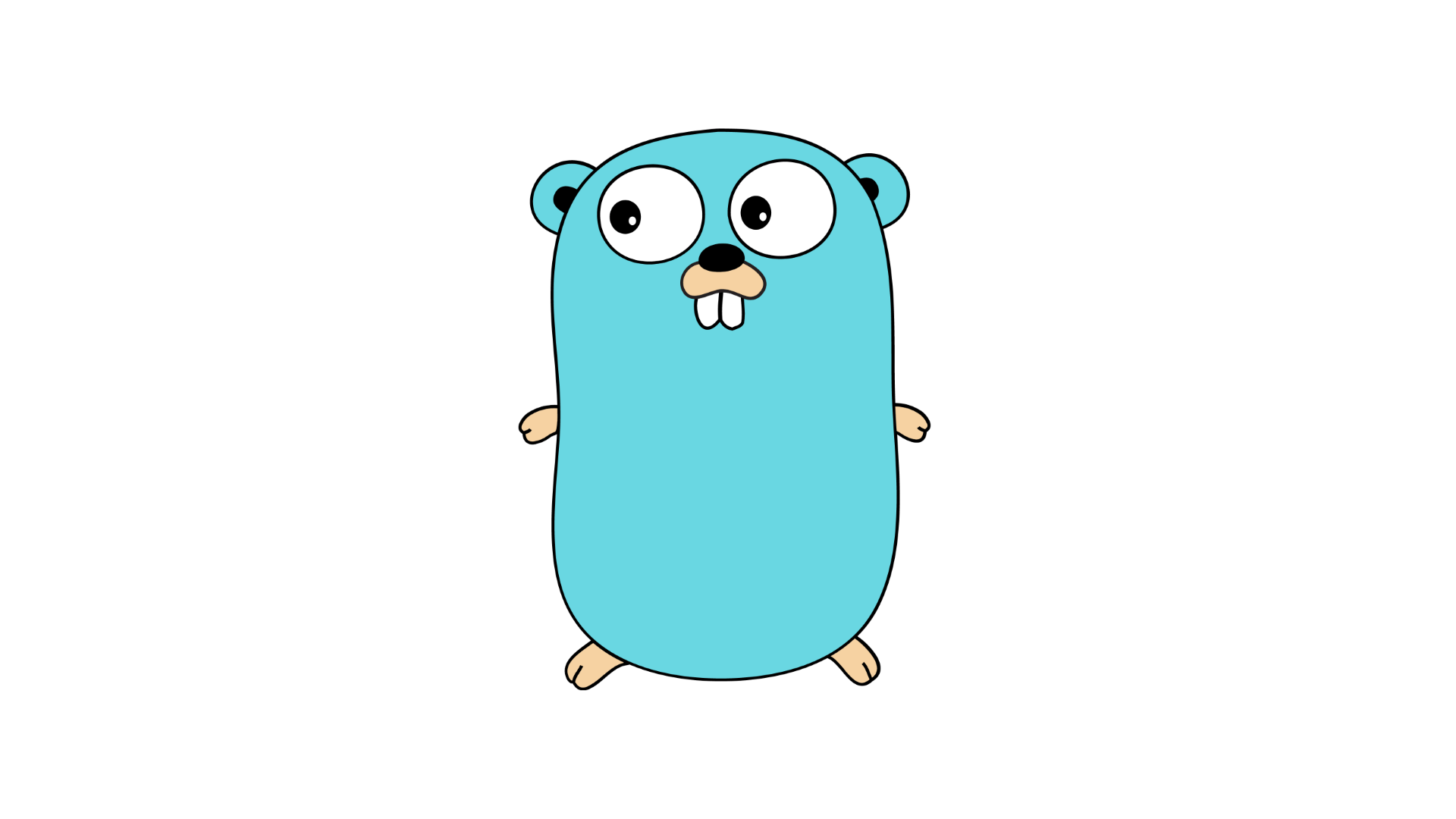
Introduction
Error handling is a critical aspect of writing robust and reliable software applications. In Go (Golang), handling errors effectively is even more important due to the language's explicit error handling approach. In this blog post, we will explore the best practices, principles, and patterns for error handling in Go, providing you with valuable insights and techniques to write error-free code.
Error Handling in Go: Principles
Principle 1: Errors are Values
In Go, errors are treated as values rather than exceptions. This enables a clean and concise error handling approach, where errors can be checked explicitly. We'll dive into the philosophy behind errors as values in Go and learn how to treat errors as first-class citizens in our code.
Principle 2: Fail Early and Explicitly
One of the core principles of Go error handling is failing fast and explicitly. This means that when an error occurs, it should be handled as close to the source of the error as possible. We'll explore the concept of failing fast and discuss the benefits of explicit error handling.
Principle 3: Provide Sufficient Context
Providing meaningful error messages and sufficient context is crucial for effective error handling. We'll delve into the importance of context in error messages and explore techniques for adding relevant information to errors that can aid in debugging and troubleshooting.
Patterns for Error Handling
Pattern 1: Error Type Assertion
When dealing with specific types of errors, using type assertions can be beneficial. We'll learn how to use type assertions to handle different types of errors and provide code samples that demonstrate their usage in practical scenarios.
Pattern 2: Error Wrapping
Error wrapping is a technique that allows us to add additional information to errors without losing the original error context. We'll explore the concept of error wrapping, understand its advantages, and discuss best practices for implementing error wrapping in Go code.
Pattern 3: Error Handling Functions
Implementing dedicated error handling functions can improve code readability and maintainability. We'll introduce the concept of error handling functions and discuss the benefits and best practices for implementing them in our code.
Best Practices for Error Handling
Use Errors.As
The Errors.As function in Go can be used to unwrap and handle specific types of errors. We'll learn how to leverage the Errors.As function effectively and provide code examples that demonstrate its usage for better error handling.
Implement Error Wrapping
Implementing error wrapping properly is key to maintaining error context and effective error propagation. We'll discuss guidelines for implementing error wrapping in Go code, explore trade-offs, and consider various aspects to ensure our error wrapping is efficient and maintainable.
Utilize Context Package
The context package in Go provides a powerful mechanism for propagating errors across multiple goroutines. We'll explore the importance of the context package in error handling, understand its role in error propagation, and discuss the benefits of using context in our code.
Error Handling in Concurrent Applications
Dealing with Goroutine Errors
Error handling in concurrent applications introduces unique challenges. We'll discuss the challenges of error handling in Goroutines and explore techniques for effectively handling errors in concurrent code. Code samples will be provided to illustrate these techniques.
Error Aggregation and Reporting
Aggregating, reporting, and logging errors in a concurrent environment requires careful consideration. We'll discuss strategies for aggregating and reporting errors in concurrent programs and provide best practices for monitoring and analyzing errors to ensure the stability and reliability of our concurrent applications.
Conclusion
Congratulations on exploring the best practices, principles, and patterns for error handling in Go! By adopting these practices, you'll be able to write more robust and reliable code, handle errors effectively, and ensure the stability of your applications.
Remember, error handling is an essential part of software development, and mastering it will significantly improve the quality of your Go code. Keep practicing these principles and patterns, and you'll become more proficient in writing error-free code in Go.
Stay tuned for more informative blog posts where we'll continue to explore advanced topics in Go programming. Happy coding!