Zero Allocation in Golang: Boosting Speed and Efficiency
Learn how to achieve zero allocation in Go (Golang) by avoiding unnecessary allocations, using pointers, and leveraging escape analysis for stack allocation. Boost the speed and efficiency of your programs.
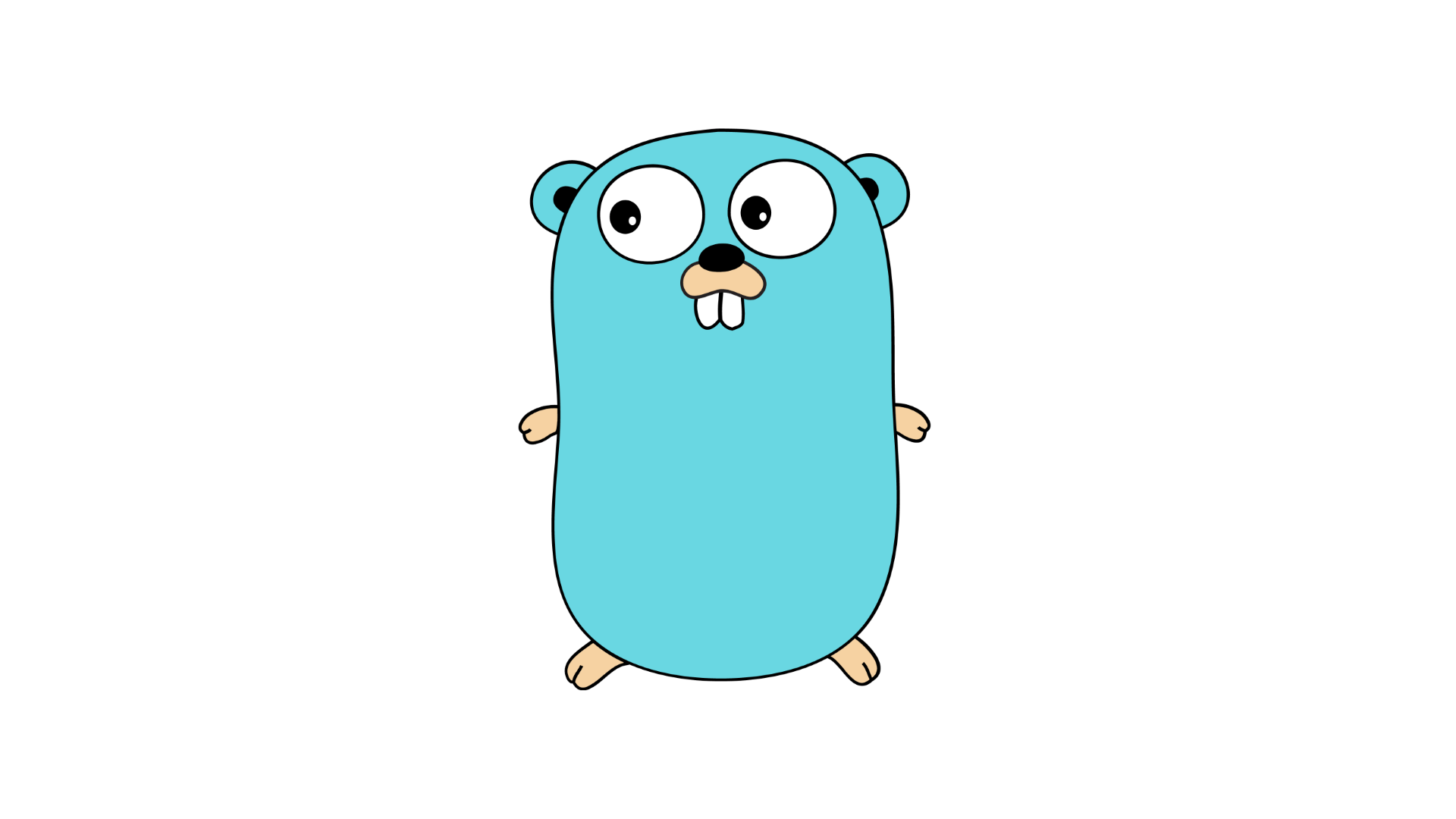
Introduction
As a beginner in Go (Golang) development, you may have heard about the importance of minimizing memory allocations for improving performance. In this blog post, we will explore the concept of zero allocation in Go and how it can boost the speed and efficiency of your programs.
What Is Allocation?
In Go, allocation refers to the process of reserving memory for storing variables, data structures, and other program entities. Whenever we create a new variable or data structure, the Go runtime allocates memory to store its value. While memory allocation is essential for program execution, excessive allocations can have a negative impact on performance.
The Cost of Allocation
Allocations in Go are not free. They involve CPU cycles and memory management overhead. Frequent allocations can cause unnecessary memory fragmentation and garbage collection overhead, leading to slower program execution.
When you allocate memory in Go, the memory is allocated on the heap. The heap is a region of memory managed by the Go runtime, and it is where dynamically allocated memory resides. The Go runtime also provides a garbage collector that automatically reclaims unused memory.
Zero Allocation in Golang
The concept of zero allocation in Go refers to minimizing or eliminating unnecessary memory allocations during program execution. By reducing allocations, you can significantly improve the performance and efficiency of your programs.
Avoiding Unnecessary Allocations
One way to achieve zero allocation in Go is by avoiding unnecessary allocations in the first place. Here are a few techniques:
- Reuse Data Structures: Instead of creating a new data structure for each operation, consider reusing existing data structures. Reusing data structures eliminates the need for frequent allocations and improves performance.
- Preallocate Slices and Maps: If you know the maximum size of a slice or map in advance, you can preallocate the required capacity instead of relying on the default behavior, which involves resizing and reallocating memory as needed.
- Buffer Pooling: Buffer pooling is a technique where you maintain a pool of reusable buffer objects. Instead of allocating new buffers each time, you can borrow buffers from the pool and return them when they are no longer needed. This technique is especially useful when working with I/O operations.
Using Pointers and Pointers to Slices
Another way to minimize allocations is by using pointers and pointers to slices. Instead of passing values by copying them, you can pass pointers to the values or slices. This way, you avoid the need to allocate new memory for each copy, resulting in more efficient memory usage.
Be careful when using pointers, as they can introduce complexity and potential issues, such as accessing already freed memory. Use pointers judiciously and make sure to manage the lifetime of allocated memory properly.
Stack Allocation with Escape Analysis
Go's escape analysis plays a crucial role in achieving zero allocation. The escape analysis determines whether a variable or value escapes the current function stack frame. If the variable or value does not escape, the Go compiler can allocate it on the stack instead of the heap. Stack allocations are faster and have lower overhead compared to heap allocations.
To help the escape analysis, follow these guidelines:
- Limit the use of global variables: Global variables are likely to escape the stack frame, resulting in heap allocations.
- Avoid creating closures: Closures can lead to heap allocation if they capture variables that need to outlive the current function.
- Avoid passing variables by capturing references: When passing variables to a function as arguments, avoid capturing references unnecessarily.
Benchmarking Zero Allocation
When optimizing for zero allocation, it's essential to measure the impact of your changes. Go provides a built-in benchmarking package (testing
) and a benchmarking tool (go test -bench
) that allow you to write and run benchmarks to measure the performance of your code.
By comparing the performance and memory usage of different implementations, you can determine the effectiveness of your zero allocation techniques and make informed decisions about their usage in your codebase.
Wrapping Up
Minimizing memory allocation is an essential aspect of writing performant and efficient Go code. By reducing unnecessary allocations through techniques such as reuse, preallocation, and buffer pooling, as well as utilizing pointers and the escape analysis, you can significantly improve the speed and efficiency of your programs.
Remember, optimizing for zero allocation requires a balance between performance and maintainability. Make sure to benchmark your code and validate the improvements before applying these techniques at scale.
Now that you understand the concept of zero allocation in Go, try applying these techniques in your own projects and observe the improvements in performance and efficiency. Happy coding!