Applying Domain-Driven Design to Real-World Golang Projects: Case Studies and Examples
Learn how to apply Domain-Driven Design (DDD) principles to your Golang projects. Explore case studies, examples, and practical techniques for building robust and maintainable software systems.
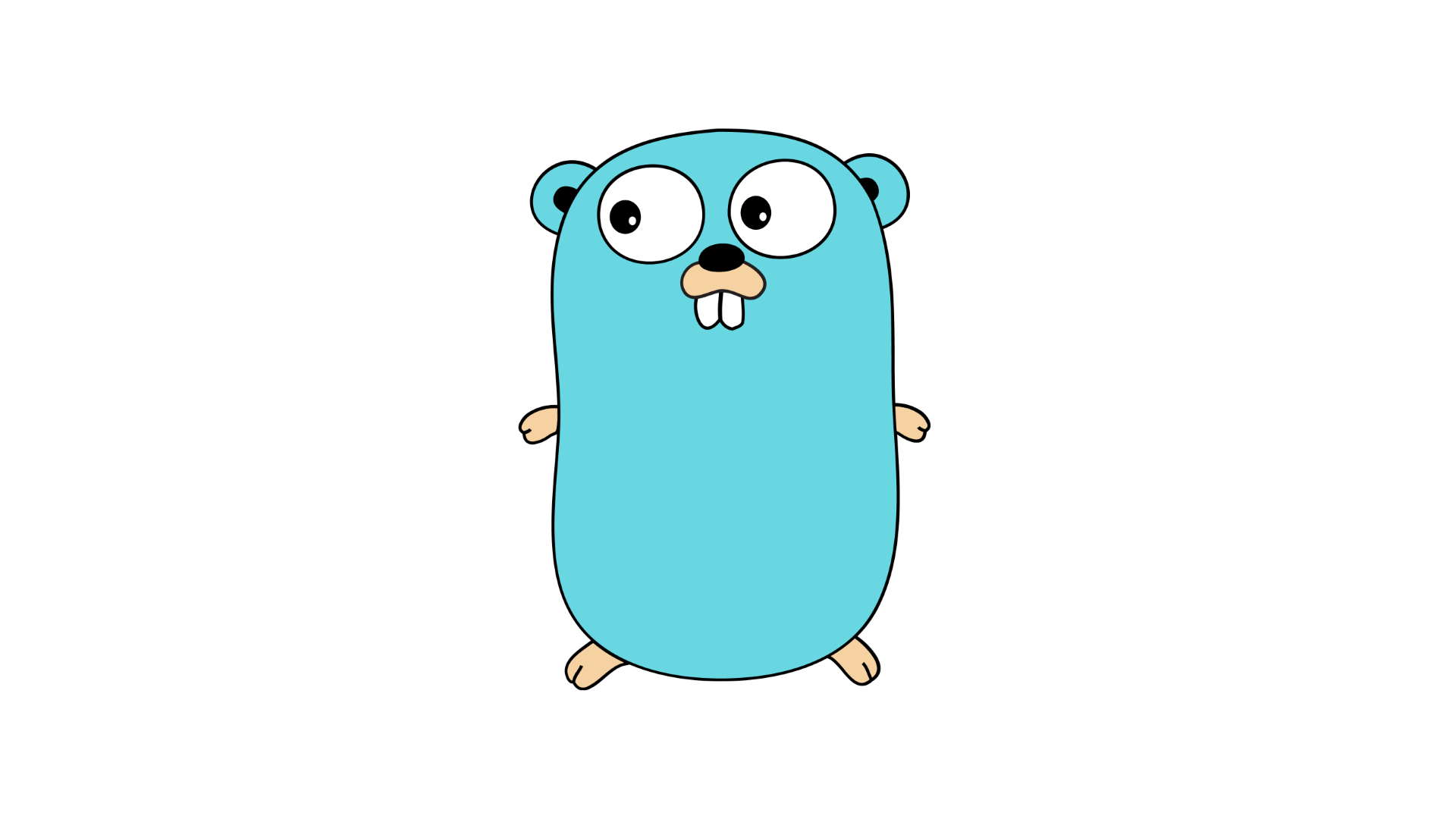
Applying Domain-Driven Design to Real-World Golang Projects: Case Studies and Examples
Welcome to our comprehensive guide on applying Domain-Driven Design (DDD) to real-world Golang projects! In this article, we'll dive into the principles and concepts of DDD and explore how they can be implemented in Golang. We'll also provide you with case studies and examples to help you understand how DDD can be applied to solve common problems in software development.
Introduction to Domain-Driven Design
Domain-Driven Design is an approach to software development that focuses on creating software systems that closely align with the business domain they are built for. DDD emphasizes deep understanding and modeling of the business domain, enabling developers to create well-structured, maintainable, and scalable applications.
DDD introduces several key concepts and principles that help developers tackle the complexity of real-world domains:
- Ubiquitous Language: DDD emphasizes the use of a common language between developers and domain experts to ensure clear communication and shared understanding.
- Bounded Contexts: In large software projects, it's common to have different sections of the system that function independently. Bounded Contexts allow developers to divide the system into smaller, more manageable parts.
- Aggregates: Aggregates are a set of closely related objects that are treated as a single unit. They encapsulate business rules and ensure consistency within the domain.
- Entities: Entities represent long-lived objects that have a unique identity. They are responsible for maintaining their state and enforcing invariants.
- Value Objects: Value Objects are objects that carry attributes without an identity. They are immutable and play a crucial role in expressing concepts and constraints within the domain.
- Domain Events: Domain Events capture an important occurrence within the system. They are used to communicate changes and trigger actions in other parts of the system.
Applying Domain-Driven Design in Golang Projects
Now that we have a basic understanding of DDD, let's explore how we can apply these principles and concepts in Golang projects.
Modeling the Domain with Structs
In Golang, we can model the domain using structs. Each struct represents an entity, aggregate, or value object in the business domain. Let's take a look at an example:
type Product struct {
ID int
Name string
Description string
Price float64
// ... other attributes
}
In this example, we have a Product struct that represents a product in an e-commerce domain. The struct contains attributes such as ID, Name, Description, and Price. By using structs, we can easily define the structure and behavior of entities within the domain.
Structuring the Project with Bounded Contexts
Golang projects can quickly become complex, with multiple components and services interacting with each other. To manage this complexity, we can apply the concept of Bounded Contexts.
Each Bounded Context represents a specific section of the system and has its own models, services, and repositories. This ensures that each part of the system is focused on its own core functionality and encapsulates its own business logic.
For example, in a blogging platform, we might have separate Bounded Contexts for handling user authentication, managing blog posts, and tracking analytic data. Each Bounded Context would have its own set of models, services, and repositories specifically tailored to its needs.
Implementing Aggregates, Entities, and Value Objects
In Golang, we can implement aggregates, entities, and value objects using a combination of structs and methods.
An aggregate, as mentioned earlier, is a set of closely related objects. Let's see how we can implement an aggregate in Golang:
type Order struct {
ID int
CustomerID int
LineItems []LineItem
// ... other attributes
}
type LineItem struct {
ProductID int
Quantity int
}
In this example, the Order struct represents an aggregate containing LineItem structs. The Order struct encapsulates business rules related to managing orders, while the LineItem struct represents the relationship between the Order and the Product.
We can also define entities and value objects using structs:
type User struct {
ID int
Name string
Email string
Password []byte
// ... other attributes
}
type Money struct {
Amount float64
Currency string
}
In this example, the User struct represents an entity with unique identification, and the Money struct represents a value object that holds an amount and a currency.
Event-Driven Architecture with Domain Events
Domain Events are an essential part of DDD, and they can be implemented in Golang using different patterns and techniques. One popular approach is to use a pub/sub mechanism to communicate and handle events within the system.
There are several libraries and frameworks available in Golang that can help you implement an event-driven architecture, such as NATS, RabbitMQ, or Apache Kafka. These technologies allow you to publish and subscribe to domain events, enabling loose coupling and asynchronous communication between different parts of your system.
Real-World Case Studies
To better understand how DDD can be applied in real-world Golang projects, let's explore a couple of case studies:
Case Study 1: Building a Car Rental Service
In this case study, we'll consider a hypothetical car rental service. We can identify several domains in this project, including user management, car inventory, and rental bookings.
By applying DDD principles, we can model each domain using well-defined structs and separate them into different Bounded Contexts. For example:
- User Management Bounded Context: Manages user authentication, registration, and profile information.
- Car Inventory Bounded Context: Handles car management, including adding new cars to the inventory and tracking their availability.
- Rental Bookings Bounded Context: Deals with the rental booking process, including reservation requests and payment handling.
By implementing these Bounded Contexts and modeling the entities, aggregates, and value objects within each context, we can create a well-structured and maintainable car rental service.
Case Study 2: Creating a Chat Application
In this case study, let's consider a chat application where users can send messages and join different chat rooms.
Using DDD, we can divide the chat application into several Bounded Contexts:
- User Management Bounded Context: Manages user authentication, registration, and profile information, including online status and friend lists.
- Chat Room Bounded Context: Handles chat room creation, joining/leaving chat rooms, and sending/receiving messages.
By modeling each Bounded Context and its corresponding entities, aggregates, and value objects, we can build a scalable and maintainable chat application.
Conclusion
Domain-Driven Design is a powerful approach that allows developers to build robust, scalable, and maintainable software systems. By modeling the domain using structs, dividing the project into Bounded Contexts, implementing aggregates, entities, and value objects, and leveraging domain events, we can create well-structured Golang projects that closely align with the business domain they are built for.
In this article, we explored the principles and concepts behind DDD, along with practical examples and case studies. By applying DDD to real-world Golang projects, you can enhance the quality and maintainability of your software solutions.
Stay tuned for our next article, where we'll explore advanced DDD techniques and best practices in Golang development. Happy coding!