Applying Domain-Driven Design Patterns in Golang Projects
Domain-driven design (DDD) in Golang: Learn how to align business requirements with the software design, leverage DDD patterns like aggregates, repositories, factories, services, value objects, and event sourcing for more maintainable and scalable applications.
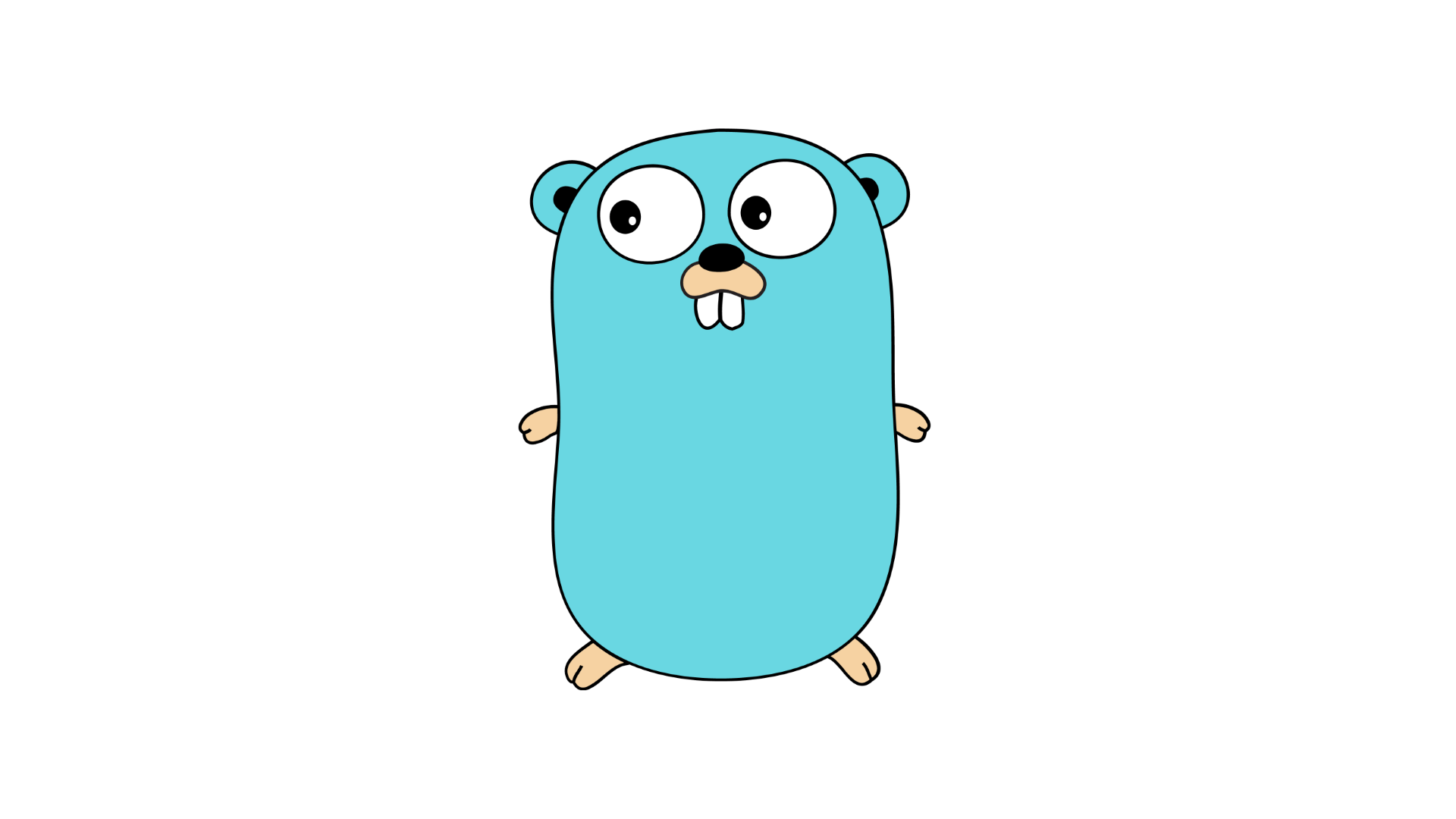
Introduction
Domain-driven design (DDD) is a software development approach that focuses on aligning business requirements with the software design. By placing the domain at the core of the application, DDD aims to create maintainable and scalable software systems. In this article, we will explore how to apply DDD patterns in Golang projects, leveraging the language's simplicity and powerful features.
Why is Domain-Driven Design Important?
Domain-driven design is crucial for building complex and scalable applications. By focusing on the business domain, DDD helps to:
- Clear Communication: DDD promotes a shared language between domain experts and developers, leading to effective communication and a better understanding of business requirements.
- Better Software Design: With DDD, the software design aligns closely with the business domain, resulting in a more maintainable and flexible codebase.
- Scalability: By decoupling the domain logic from infrastructure concerns, DDD enables the application to scale more effectively.
Applying Domain-Driven Design Patterns in Golang Projects
Let's explore some of the key DDD patterns and how they can be applied in Golang projects:
1. Aggregate Root
In DDD, an aggregate is a cluster of domain objects that forms a transactional consistency boundary. The aggregate root is the main entity within the aggregate that acts as the access point for the outside world. In Golang, an aggregate root can be represented as a struct containing the related domain objects.
```go type Order struct { ID int Customer string Total float64 Status string // ... other fields and methods } ```
2. Repository
The repository pattern provides an abstraction layer between the domain model and the persistence layer. It encapsulates all the logic required for data access, separation of concerns, and improves testability. In Golang, we can implement the repository pattern using interfaces and dependency injection.
```go type OrderRepository interface { Save(order *Order) error GetByID(id int) (*Order, error) // ... other repository methods } ``` ```go type OrderRepositoryImpl struct { // ... implementation details } func (r *OrderRepositoryImpl) Save(order *Order) error { // ... implementation details } func (r *OrderRepositoryImpl) GetByID(id int) (*Order, error) { // ... implementation details } ```
3. Factory
The factory pattern is used to create complex objects. It encapsulates the object creation logic and provides a consistent way to create objects throughout the application. In Golang, we can implement the factory pattern using functions that return the desired objects.
```go func NewOrder(customer string, total float64) *Order { return &Order{ Customer: customer, Total: total, Status: "Created", } } ```
4. Service
Service objects encapsulate the behavior that does not belong to any entity or value object. They coordinate operations across multiple entities and are responsible for enforcing business rules. In Golang, we can create service objects as standalone functions or by grouping related functions in a struct.
```go type OrderService struct { OrderRepo OrderRepository // ... other dependencies } func (s *OrderService) CreateOrder(customer string, total float64) (*Order, error) { order := NewOrder(customer, total) // ... perform business logic if err := s.OrderRepo.Save(order); err != nil { return nil, err } return order, nil } ```
5. Value Object
A value object represents a concept within the domain that does not have an identity. Value objects are immutable and give meaning to the attributes of an entity. In Golang, we can create value objects as structs with value-based equality and immutable fields.
```go type Money struct { Amount float64 Currency string // ... methods } ```
6. Event Sourcing
Event sourcing is a technique for persisting the state of an application by storing a sequence of events. These events can be replayed to reconstruct the state of any point in time. In Golang, we can use event sourcing to implement event-driven architectures and ensure data consistency.
```go type Event struct { AggregateID int EventType string EventData string // ... other fields } func (e *Event) Apply() error { // ... apply event logic } ```
Summary
Applying domain-driven design patterns in Golang projects can lead to more maintainable, scalable, and business-aligned software systems. By leveraging concepts like aggregates, repositories, factories, services, value objects, and event sourcing, we can create software that truly represents the business domain.
Remember, DDD is not a one-size-fits-all approach, but understanding and applying its core principles can greatly benefit your projects.
Happy coding!