Domain-Driven Design and Test-Driven Development in Golang: A Winning Combination
"Learn how Domain-Driven Design (DDD) and Test-Driven Development (TDD) can improve your Go projects by promoting clean code, design clarity, and refactoring friendliness."
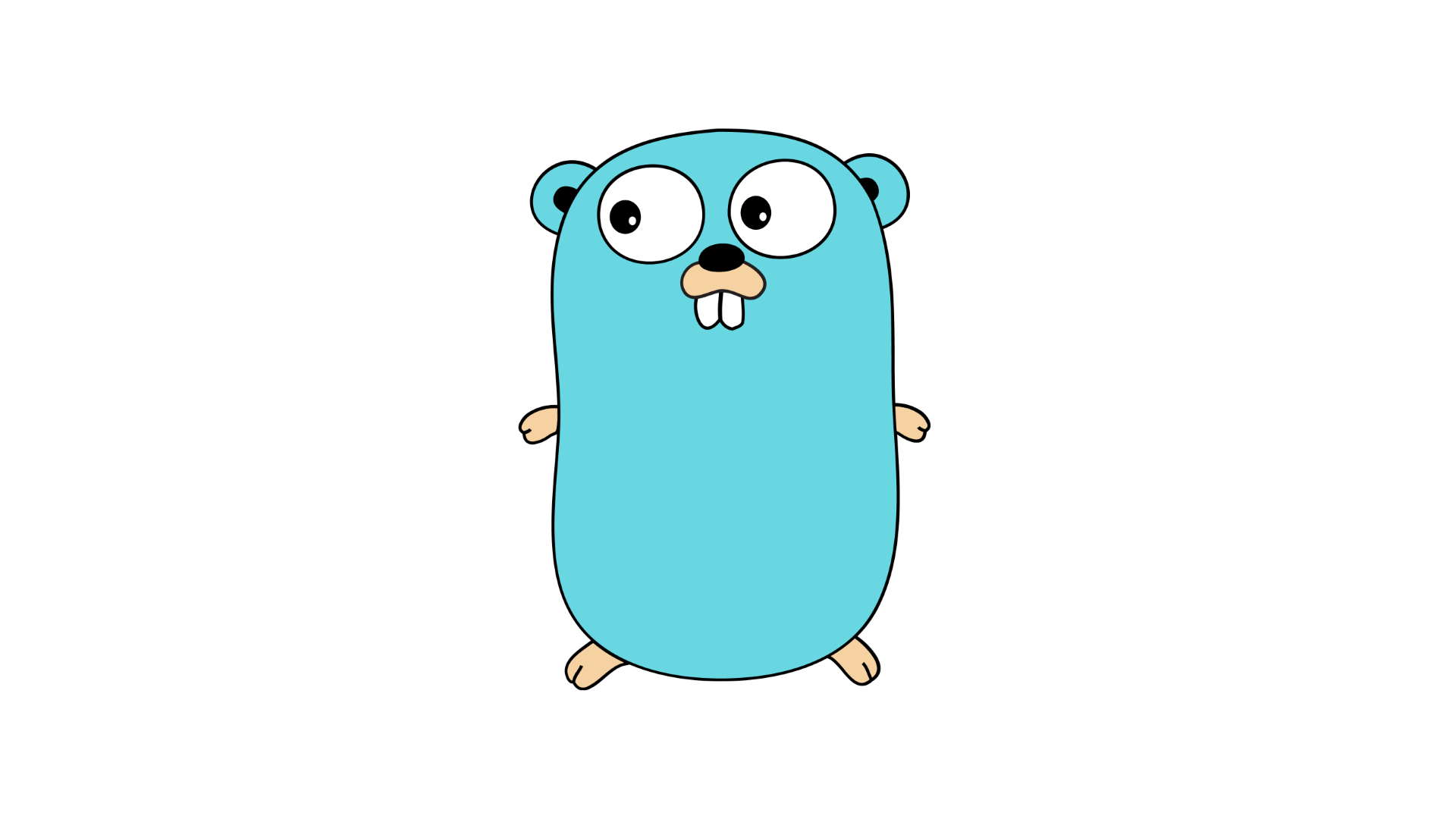
Introduction
Domain-Driven Design (DDD) and Test-Driven Development (TDD) are two powerful methodologies that can significantly improve the development process and the quality of your code. When combined, they form a winning combination that promotes clean, maintainable, and testable code. In this blog post, we'll explore how DDD and TDD can be applied in the context of Go (Golang) development.
What is Domain-Driven Design?
Domain-Driven Design is an architectural approach that focuses on modeling the domain of a software application. It emphasizes the importance of building a deep understanding of the problem domain and using that understanding to drive the design of the solution. DDD encourages the creation of well-designed, modular, and reusable code by breaking the domain into small, manageable components called bounded contexts.
In DDD, the domain is the central part of the application. It represents the core business logic and encapsulates the knowledge and rules that drive the software's behavior. By clearly defining the domain and modeling it in code, DDD enables developers to write code that closely aligns with the real-world problem it is solving.
What is Test-Driven Development?
Test-Driven Development is a software development process that relies on writing tests before writing the actual implementation code. The idea is to first write a test that defines the behavior or functionality that is expected from the code. Then, the implementation code is written with the goal of making the test pass.
By following the TDD cycle of writing a failing test, writing the implementation code, and then refactoring the code, developers can ensure that their code stays maintainable, and that it always meets the specified requirements. TDD also promotes a test-first mindset, enforcing a rigorous approach to testing and preventing bugs from entering the codebase.
The Benefits of DDD and TDD in Golang
By combining DDD and TDD in your Go projects, you can capitalize on the benefits of both methodologies and create high-quality software. Here are some advantages:
1. Code Quality
DDD and TDD both put a strong emphasis on code quality. DDD promotes the creation of clean, modular, and reusable code by modeling the domain using bounded contexts. TDD ensures that all code is thoroughly tested, leading to fewer bugs and more reliable software.
2. Design Clarity
Using DDD in Go helps you create a clear and consistent design of your software. By dividing the domain into logical components and modeling them in code, you can easily understand and communicate the structure and behavior of your application.
3. Refactoring Friendliness
TDD encourages a test-first mindset and the practice of refactoring. The combination of DDD and TDD provides a strong foundation for refactoring code. With a comprehensive test suite, you can confidently refactor your code to improve its design, performance, or any other aspect without fear of breaking features.
4. Collaboration
Both methodologies encourage collaboration among team members. DDD promotes a shared understanding of the domain, enabling developers and domain experts to work together to build the best solution. TDD ensures that the code is thoroughly tested, making it easier for team members to review and contribute to the codebase.
Applying DDD and TDD in Golang
Now that we understand the benefits, let's explore how you can apply DDD and TDD in your Go projects:
1. Define the Domain Model
In DDD, the first step is to define the domain model. Start by identifying the main entities, value objects, and aggregates that make up the domain. Then, create the corresponding Go structs to represent these concepts.
type User struct {
ID int
Name string
Email string
Address Address
}
type Address struct {
Street string
City string
Country string
}
2. Write Tests
Begin with writing tests for the behavior you want to implement. Think about the various scenarios and edge cases, and test them. By writing tests first, you ensure that the code you write is testable and focused on delivering the desired functionality.
func TestGetUserByID(t *testing.T) {
// ... Setup test data
user, err := GetUserByID(1) // Example function to retrieve a user by ID
// ... Assert the expected behavior
}
3. Implement the Code
With the tests in place, you can proceed to write the implementation code. Focus on making the tests pass while following good object-oriented design principles and the principles of DDD.
func GetUserByID(userID int) (*User, error) {
// ... Retrieve the user from the database or any other data source
return &User{
ID: userID,
Name: "John Doe",
Email: "[email protected]",
Address: Address{
Street: "123 Main St",
City: "New York",
Country: "USA",
},
}, nil
}
4. Refactor
After the code is implemented and the tests pass, you can refactor the code to improve its design, performance, or any other aspect. The comprehensive test suite gives you the confidence to refactor without fear of introducing bugs.
Conclusion
Combining Domain-Driven Design and Test-Driven Development can result in clean, modular, and maintainable code. By employing DDD, you can model the domain and promote a clear and consistent design. TDD ensures comprehensive test coverage, leading to fewer bugs and more reliable code.
When applying DDD and TDD in Go projects, focus on defining the domain model, writing tests first, implementing the code, and continuously refactoring. By following this approach, you can build high-quality software that accurately reflects the problem domain and delivers the desired functionality.
Give it a try in your next Go project, and experience the benefits of this winning combination firsthand!