Apache Kafka Consumer API: Reading Data from Kafka Topics
Learn how to consume data from Kafka topics using the Kafka Consumer API in Java and Python. Explore key components like consumer groups, topics, partitions, and offsets. Build scalable and fault-tolerant data processing applications with Apache Kafka.
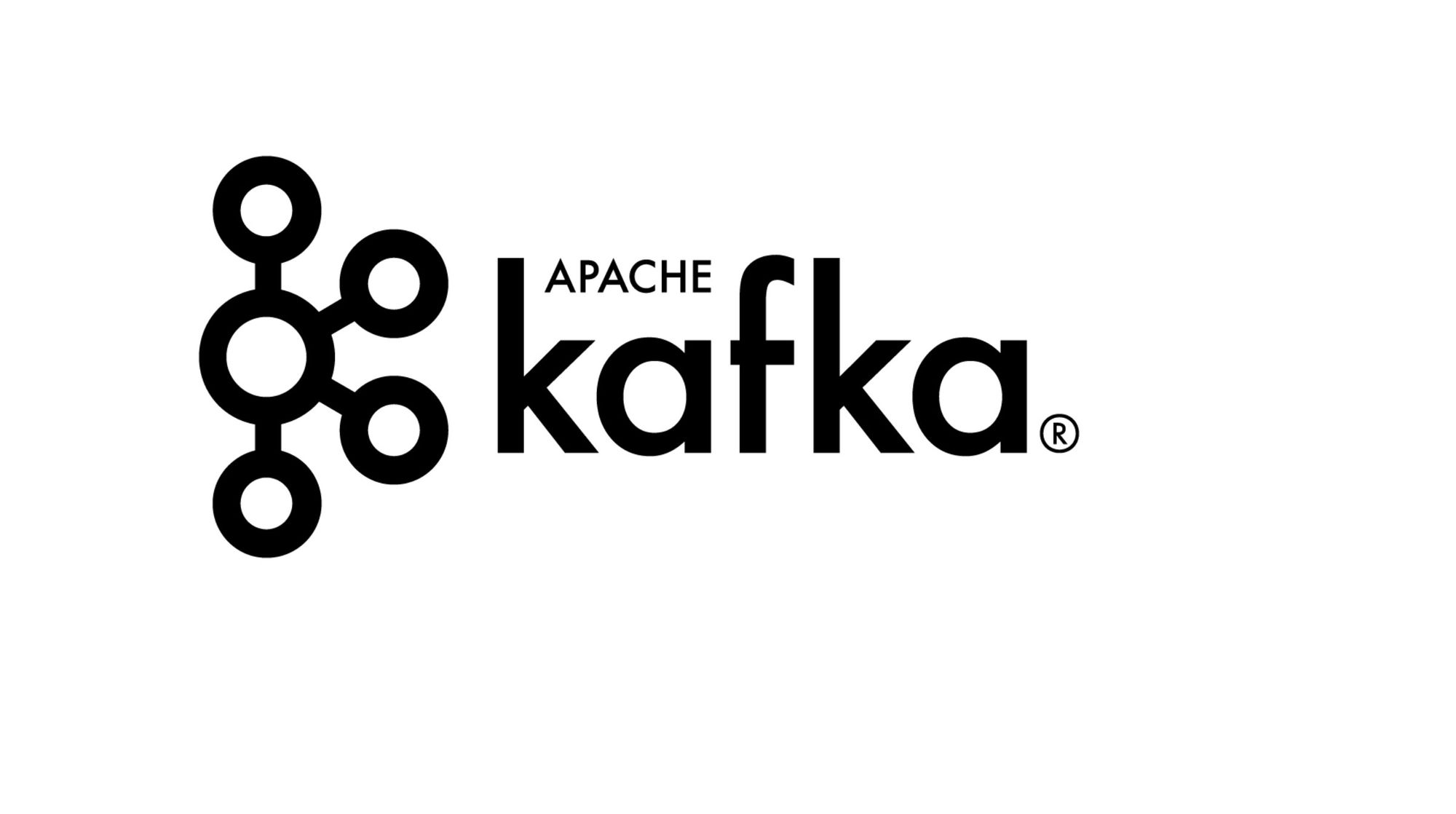
Introduction
Apache Kafka is a highly scalable and distributed streaming platform that enables efficient data ingestion, processing, and analysis in real-time. One of the core components of Kafka is the Consumer API, which allows applications to read data from Kafka topics and process it as needed. In this blog post, we'll explore the Apache Kafka Consumer API and learn how to consume data from Kafka topics using both the Java and Python programming languages. Let's get started!
Overview of the Kafka Consumer API
The Kafka Consumer API provides a convenient way for applications to read data from Kafka topics. It offers a simple and flexible programming interface, allowing developers to consume data in a variety of ways. Here are the key components of the Kafka Consumer API:
Consumer Groups
A consumer group is a group of consumers that work together to consume data from Kafka topic partitions. Each consumer group can have multiple consumer instances, and each instance is responsible for consuming data from one or more partitions. Consumer groups provide scalability and fault tolerance, as multiple consumers can work in parallel to process data from different partitions.
Topics and Partitions
A Kafka topic is a category or feed into which producers write data, and from which consumers read data. Topics are divided into partitions, each of which is an ordered and immutable sequence of records. The number of partitions determines the parallelism of data consumption, as each partition can be consumed by only one consumer instance at a time.
Offsets
Each record in a partition has a unique offset, which represents its position in the partition. Offsets provide a way for consumers to track their progress, as they can commit the offsets of processed records and continue from where they left off in case of failures or restarts. The Consumer API also allows for manually specifying the offset from which to start consuming data.
Rebalancing
Rebalancing occurs when consumers join or leave a consumer group, or when new partitions are added or removed from a topic. During rebalancing, Kafka automatically and evenly distributes the partitions across the available consumer instances, ensuring that each instance is responsible for consuming data from a fair share of partitions.
Consuming Data from Kafka Topics in Java
Now let's look at how we can consume data from Kafka topics using the Kafka Consumer API in Java. Here are the steps involved:
1. Include the Kafka Clients Dependency
First, you'll need to add the Kafka Clients dependency to your Maven or Gradle project. Add the following dependency to your build file:
```xml org.apache.kafka kafka-clients ${kafka.version}``` Replace `${kafka.version}` with the desired Kafka version. This dependency includes the necessary classes for consuming data from Kafka topics.
2. Create a Kafka Consumer
Next, you'll need to create an instance of the KafkaConsumer class. The KafkaConsumer takes key-value pairs representing the configuration properties as arguments. Here's an example:
```java Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("group.id", "my-consumer-group"); props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); KafkaConsumer consumer = new KafkaConsumer<>(props); ``` In this example, we specify the bootstrap servers (comma-separated list of kafka brokers), the consumer group id, and the key and value deserializers for reading the records from Kafka. You can customize the consumer configuration properties based on your requirements.
3. Subscribe to Kafka Topics
After creating a KafkaConsumer instance, you can subscribe to one or more Kafka topics using the subscribe method. Here's an example: ```java consumer.subscribe(Arrays.asList("topic1", "topic2")); ``` In this example, we subscribe to two topics: "topic1" and "topic2". The consumer will then start fetching data from these topics.
4. Consume Records
Once you have subscribed to the topics, you can start consuming records from Kafka using the poll method. The poll method returns a batch of records, which you can then process as needed. Here's an example: ```java while (true) { ConsumerRecords records = consumer.poll(Duration.ofMillis(100)); for (ConsumerRecord record : records) { System.out.println(record.key() + ": " + record.value()); } } ``` In this example, we continuously poll for records every 100 milliseconds. For each batch of records, we iterate over them and print the key and value of each record. You can replace the print statement with your own logic to process the records.
5. Committing Offsets
If you want to manually commit the offsets of processed records, you can call the commitSync or commitAsync methods on the consumer instance. Committing offsets allows the consumer to track its progress and continue from where it left off in case of failures or restarts. ```java consumer.commitSync(); ``` or ```java consumer.commitAsync(); ```
Consuming Data from Kafka Topics in Python
In addition to Java, you can also consume data from Kafka topics using the Kafka Consumer API in Python. Here's how you can do it:
1. Install the Kafka Python Library
First, you'll need to install the kafka-python library, which provides Python bindings for the Kafka Consumer API. You can install it using pip: ``` pip install kafka-python ```
2. Create a Kafka Consumer
Next, you'll need to create an instance of the KafkaConsumer class. The KafkaConsumer takes key-value pairs representing the configuration properties as arguments. Here's an example: ```python from kafka import KafkaConsumer consumer = KafkaConsumer( "topic", bootstrap_servers="localhost:9092", group_id="my-consumer-group", value_deserializer=lambda x: x.decode("utf-8") ) ``` In this example, we specify the bootstrap servers (comma-separated list of kafka brokers), the consumer group id, and the value deserializer for reading the records from Kafka. You can customize the consumer configuration properties based on your requirements.
3. Consume Records
Once you have created a KafkaConsumer instance, you can start consuming records from Kafka using the poll method. The poll method returns a batch of records, which you can then process as needed. Here's an example: ```python for record in consumer: print(record.key, record.value) ``` In this example, we iterate over the records returned by the poll method and print the key and value of each record. You can replace the print statement with your own logic to process the records.
4. Committing Offsets
If you want to manually commit the offsets of processed records, you can call the commit method on the consumer instance. Committing offsets allows the consumer to track its progress and continue from where it left off in case of failures or restarts. ```python consumer.commit() ```
Conclusion
The Apache Kafka Consumer API provides a powerful and flexible way to consume data from Kafka topics. Whether you're using Java or Python, you can easily read data from Kafka and process it according to your application's needs. By understanding the key components of the Kafka Consumer API and following the steps outlined in this blog post, you'll be well-equipped to consume data from Kafka topics and build scalable and fault-tolerant data processing applications. Happy consuming!