Angular Routing: Creating Single-Page Applications with Ease
Routing in Angular allows you to create single-page applications with ease, providing seamless navigation between views. Learn how to set up routing and navigate between routes in Angular for a smooth user experience.
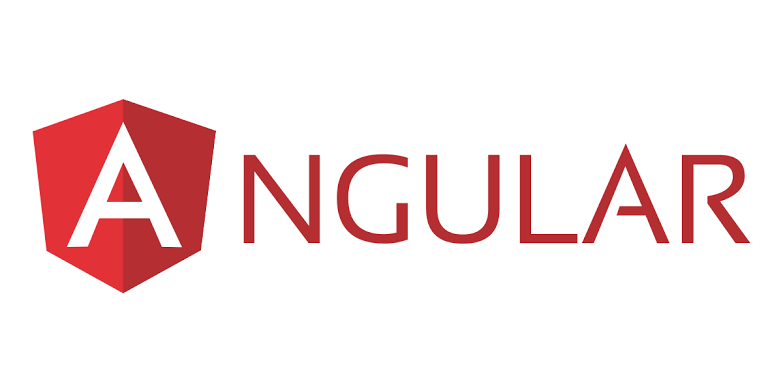
Introduction
Angular is a popular JavaScript framework for building dynamic web applications. One of its powerful features is routing, which allows you to create single-page applications (SPAs) with ease. By implementing routing in your Angular project, you can navigate between different views without refreshing the entire page, providing a seamless and interactive user experience.
What is Routing in Angular?
Routing in Angular refers to the process of determining which component should be loaded based on the URL, allowing you to create multiple views within a single page. These views are managed by Angular's Router module, which provides a way to map URLs to specific components.
Angular's routing works by defining routes that specify which components should be loaded for specific URLs. When a user navigates to a certain route, Angular uses the router configuration to determine which component to load and displays it in the designated area of the page.
Setting Up Routing in Angular
To start using routing in your Angular project, you need to:
1. Install the Router module
Before you can use routing, you need to install the Router module. You can do this by running the following command in your terminal:
npm install @angular/router
2. Define Routes
Once the Router module is installed, you can define your routes in the app-routing.module.ts file. This file is located in the src/app directory.
In this file, you'll need to import the necessary modules and define an array of routes. Each route object should have a path, which represents the URL, and a component, which specifies the component to be loaded for that URL.
Here's an example of how to define routes:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { HomeComponent } from './home.component';
import { AboutComponent } from './about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
3. Add the Router Outlet
The next step is to add the Router Outlet component to your app component. The Router Outlet acts as a placeholder where Angular can load the appropriate component based on the current route.
In your app.component.html file, add the following code:
<router-outlet></router-outlet>
4. Navigate Between Routes
With routing set up, you can now navigate between different routes using Angular's RouterLink directive. The RouterLink directive is used to create links to different routes in your application.
Here's an example of how to use the RouterLink directive:
<a routerLink="">Home</a>
<a routerLink="about">About</a>
When a user clicks on the Home link, the HomeComponent will be loaded. Likewise, when the user clicks on the About link, the AboutComponent will be loaded.
Additional Routing Features
1. Route Parameters
You can pass parameters to routes by using route parameters. Route parameters allow you to pass dynamic values in the URL and retrieve them in the component.
Here's an example of how to define a route with a parameter:
{ path: 'product/:id', component: ProductComponent }
In the example above, the :id is a route parameter that can hold any value. To access this parameter in the ProductComponent, you can use the ActivatedRoute service.
2. Nested Routes
Angular routing also supports nested routes, where you can have child routes within a parent route. This allows you to create more complex navigation structures.
To create nested routes, you need to define child routes within the parent route's configuration. Here's an example:
const routes: Routes = [
{ path: 'dashboard', component: DashboardComponent, children: [
{ path: 'users', component: UsersComponent },
{ path: 'orders', component: OrdersComponent },
]},
];
In the example above, the DashboardComponent acts as the parent route, and the UsersComponent and OrdersComponent are the child routes. When a user navigates to /dashboard/users, the UsersComponent will be loaded.
Conclusion
Routing in Angular allows you to create single-page applications with ease by providing seamless navigation between different views. By following the steps outlined in this article, you can set up routing in your Angular project and take advantage of its powerful features.
With Angular's routing, you can create dynamic and interactive web applications that provide a smooth user experience. So go ahead, start using routing in your Angular projects and take your web development skills to the next level!