Angular Performance Optimization: Speeding Up Your App
Learn how to optimize the performance of your Angular app with techniques like lazy loading, tree shaking, OnPush change detection, virtual scrolling, and more. Improve user experience and SEO rankings.
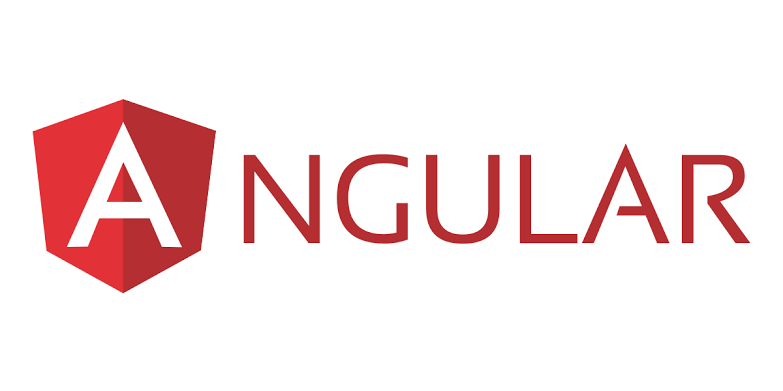
Introduction
Are you facing slow performance issues with your Angular application? Don't worry, we've got you covered! In this blog post, we'll explore various techniques and best practices for optimizing the performance of your Angular app. By implementing these strategies, you'll be able to significantly improve the speed and responsiveness of your application, resulting in a better user experience. Let's dive into the world of Angular performance optimization!
Why is Performance Optimization Important?
Performance optimization is crucial for any web application, and Angular is no exception. Slow page load times and unresponsive user interfaces can result in frustrated users and high bounce rates. Moreover, performance is a key factor in search engine rankings, so optimizing your Angular app can also help with your SEO efforts. By taking the time to optimize the performance of your application, you'll be able to deliver a smoother and more efficient user experience.
1. Analyzing the Performance of Your Angular App
Before diving into the optimization techniques, it's important to understand the current performance of your Angular app. Angular provides tools like Angular Performance Explorer that can help you analyze the performance of your application. By identifying the parts of your app that are causing performance bottlenecks, you can prioritize your optimization efforts and focus on the areas that will yield the most significant improvements.
2. Enabling Production Mode
One of the simplest ways to optimize your Angular app is to enable production mode. By default, Angular runs in development mode, which provides additional debugging and error handling capabilities. However, this comes at the cost of performance. Enabling production mode disables these additional features, resulting in a smaller bundle size and faster performance. To enable production mode, you can use the following command when running your Angular app:
ng serve --prod
In addition to enabling production mode during development, make sure to enable it when building your app for deployment. This will ensure that your production environment benefits from the performance optimizations provided by Angular.
3. Optimizing Bundle Size
The size of your application bundle directly affects the load time of your Angular app. To optimize bundle size, you can take the following steps:
3.1 Lazy Loading Modules
Lazy loading allows you to load modules asynchronously, reducing the initial load time of your application. Instead of loading all modules at once, you can load them on-demand as the user navigates through your app. To implement lazy loading, you can use the Angular Router and the loadChildren
property:
const routes: Routes = [
{ path: 'lazy', loadChildren: () => import('./lazy.module').then(m => m.LazyModule) },
];
By lazy loading modules, you can split your application into smaller chunks that can be loaded only when needed, resulting in a faster initial page load.
3.2 Removing Unused Code
Angular provides a feature called tree shaking that automatically eliminates unused code during the build process. This helps reduce the size of your application bundle. To ensure that tree shaking is enabled, make sure that you have the following configuration in your angular.json
file:
"optimization": true
In addition, take care to only import the Angular modules and libraries that are actually needed in your application. This will help eliminate unnecessary code and reduce the bundle size.
3.3 Compressing and Gzipping Assets
Compressing and gzipping your assets can significantly reduce the size of your Angular app. Angular CLI provides built-in optimization techniques like UglifyJS and Terser that automatically minify and compress your code during the build process. You can enable these optimizations by adding the following configuration in your angular.json
file:
"optimization": {
"scripts": true,
"styles": true
}
In addition to minification and compression, enabling gzip compression on your server can further reduce the size of your assets during transmission. This can be achieved by configuring your server to enable gzip compression for your Angular app's static assets.
4. Improving Change Detection
Angular's change detection mechanism can sometimes impact the performance of your app, especially when dealing with large data sets or complex UIs. Here are a few strategies to improve change detection performance:
4.1 Using OnPush Change Detection Strategy
The OnPush change detection strategy is a performance optimization technique that allows you to control when change detection is triggered. By default, Angular uses the default change detection strategy, which triggers change detection for every component on every browser event. However, with the OnPush strategy, change detection is only triggered for a component when one of its input properties changes or when an event is emitted from that component. To use the OnPush strategy, you can set the changeDetection
property in your component decorator:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ExampleComponent { }
4.2 Using Immutable Data Structures
Mutable data structures can sometimes make change detection less efficient, as Angular has to track changes in the object's properties. By using immutable data structures, you can ensure that changes are more predictable and easier to track, resulting in improved change detection performance. Libraries like Immutable.js can help you work with immutable data structures in your Angular app.
5. Optimizing Dynamic Rendering
Angular apps that render large lists or frequently updated data can benefit from optimizing dynamic rendering. Here are a few strategies to improve the performance of dynamic rendering:
5.1 Using trackBy
When rendering lists in Angular, it's important to provide a unique identifier for each item in the list. This helps Angular track individual items and update the DOM efficiently. The trackBy
function allows you to specify a unique identifier for each item:
<ng-container *ngFor="let item of items; trackBy: trackByFn">
<app-item [item]="item"></app-item>
</ng-container>
trackByFn(index: number, item: any) {
return item.id;
}
By providing a trackBy function, you can optimize the rendering of large lists and ensure that only the necessary DOM updates are performed.
5.2 Using Virtual Scrolling
Virtual scrolling is a technique that allows you to render only a subset of items that are currently visible on the screen. This can be beneficial for large lists, as it reduces the amount of DOM elements, resulting in improved performance. Angular provides the @angular/cdk
library, which includes the CdkVirtualScroll
directive that you can use to implement virtual scrolling in your Angular app.
6. Optimizing Network Requests
Reducing the number and size of network requests is crucial for optimizing the performance of your Angular app. Here are a few strategies to achieve this:
6.1 Bundling API Requests
If your app makes multiple API requests, you can optimize performance by bundling them into a single request. This can be achieved by using server-side technologies like GraphQL or by creating a dedicated API endpoint that returns the combined data.
6.2 Caching API Responses
Caching API responses can help reduce the number of network requests and improve the responsiveness of your Angular app. You can implement client-side caching using techniques like local storage or session storage, or leverage server-side caching mechanisms provided by your backend technology.
6.3 Implementing Paginated Data Loading
If your app displays large data sets, consider implementing paginated data loading. Instead of loading all data at once, you can fetch data in smaller chunks and load additional data as the user scrolls or navigates through your app. This can help reduce initial load times and improve overall performance.
7. Optimizing Pipe Performance
Pipes are a powerful feature in Angular, but they can impact performance if not used correctly. Here are a few tips for optimizing pipe performance:
7.1 Using Pure Pipes
Pipes in Angular can be either impure or pure. Impure pipes are re-evaluated on every change detection cycle, even if the input hasn't changed. Pure pipes, on the other hand, are only re-evaluated if the input changes. By default, Angular pipes are impure, so it's important to mark them as pure explicitly if their behavior doesn't depend on mutable state:
@Pipe({
name: 'purePipe',
pure: true
})
export class PurePipe implements PipeTransform { }
7.2 Limiting the Use of Pipe Chaining
Chaining multiple pipes together can result in decreased performance, especially if the pipes perform complex operations. Instead of chaining pipes, consider creating a custom pipe that combines the functionality of multiple pipes into one.
8. Using Browser's DevTools
Modern web browsers provide powerful developer tools that can help you analyze and optimize the performance of your Angular app. These tools include features like performance profiling, memory profiling, and network profiling. By using these tools, you can identify performance bottlenecks and make targeted optimizations to improve your app's speed and responsiveness.
9. Continuous Monitoring
Optimizing the performance of your Angular app is an ongoing process. Monitor your app's performance regularly and make adjustments as needed. Set up performance monitoring tools that can help you track and analyze the performance metrics of your app over time. By continuously monitoring and optimizing your app's performance, you can ensure a smooth and efficient user experience.
Wrapping Up
Congratulations on completing this comprehensive guide to Angular performance optimization! By implementing the techniques and best practices discussed in this blog post, you'll be able to significantly improve the speed and responsiveness of your Angular app. Remember to analyze the performance of your app, enable production mode, optimize bundle size, improve change detection, optimize dynamic rendering, optimize network requests, optimize pipe performance, use browser's DevTools, and continuously monitor your app's performance. With these strategies in your toolbox, you'll be well-equipped to deliver a high-performance Angular application.
Now it's time to put these optimization techniques into practice and watch your Angular app fly! Happy coding!