Angular Forms: Building Dynamic and Responsive User Inputs
This tutorial explores how to build dynamic and responsive user inputs in Angular using Reactive forms. It covers setting up the project, creating a form, binding to form controls, adding dynamic form fields, implementing form validation, and handling form submission.
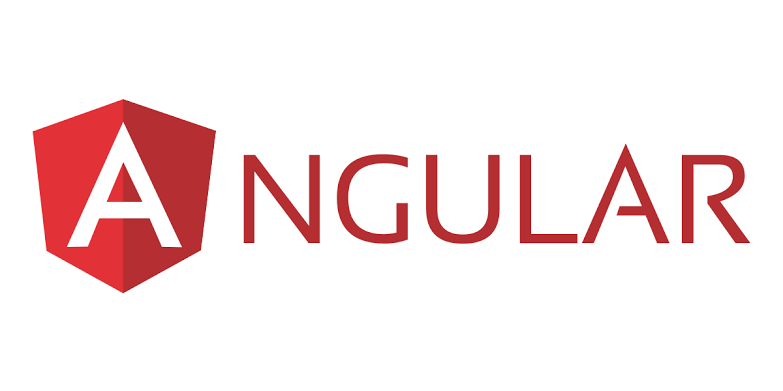
Introduction
Welcome to another exciting tutorial on Angular! In this blog post, we will delve into the world of Angular forms and learn how to build dynamic and responsive user inputs. Forms play a crucial role in web development, enabling users to provide input and interact with our applications. Angular provides a powerful and flexible way to create forms with ease.
Overview of Angular Forms
Angular offers two approaches to working with forms: template-driven forms and reactive (also known as model-driven) forms. Template-driven forms are built using Angular's template syntax, making them ideal for simple forms with basic validation requirements. Reactive forms, on the other hand, offer a more robust and flexible approach, allowing for complex forms with advanced validation and dynamic behavior. In this tutorial, we will focus on building forms using Reactive forms.
Setting Up the Project
Before we dive into building forms, let's start by setting up our project. Make sure you have Angular CLI installed. If not, run the following command to install it globally:
$ npm install -g @angular/cli
Once the installation is complete, navigate to the directory where you want to create your project and run the following command:
$ ng new angular-forms
This will create a new Angular project called "angular-forms."
Creating a Reactive Form
Let's start by creating a new component that will contain our form. Run the following command to generate a new component:
$ ng generate component form
Once the component is generated, open the form.component.html
file and add the following code:
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<label for="name">Name:</label>
<input type="text" id="name" formControlName="name">
<button type="submit">Submit</button>
</form>
In the code above, we have created a form with an input field for the user's name. We have also bound the form to a form group called "myForm" and specified a callback function (onSubmit()
) to handle form submission.
Next, open the form.component.ts
file and import the following:
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
export class FormComponent implements OnInit {
myForm: FormGroup;
constructor(private formBuilder: FormBuilder) { }
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', Validators.required]
});
}
onSubmit() {
if (this.myForm.valid) {
console.log(this.myForm.value);
}
}
}
In the code above, we have imported the necessary modules and components for form handling. We have also defined a form group called "myForm" and added a validation rule to the "name" field, making it required.
That's it! We have successfully created a reactive form in Angular. Run the following command to see the form in action:
$ ng serve
Open your browser and navigate to http://localhost:4200
. You should see the form with the input field for the user's name. Try entering a name and submitting the form. The name will be logged in the console if the form is valid.
Binding to Form Controls
In addition to basic form elements like text inputs and buttons, Angular provides a wide range of form controls that can be used to collect different types of user input. Let's explore some of the commonly used form controls.
Text Area
The <textarea>
element can be used to collect multi-line text input from the user. To use a text area in your form, update your template as follows:
<label for="message">Message:</label>
<textarea id="message" formControlName="message"></textarea>
In your component, update the form group definition to include the "message" field:
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', Validators.required],
message: ['']
});
}
Select (Dropdown)
To create a select dropdown in your form, use the <select>
element with nested <option>
elements. Update your template as follows:
<label for="gender">Gender:</label>
<select id="gender" formControlName="gender">
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select>
In your component, update the form group definition to include the "gender" field:
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', Validators.required],
message: [''],
gender: ['']
});
}
Dynamic Form Fields
Dynamic form fields allow you to create forms with a variable number of fields or fields that change based on user input. To achieve this, you can use Angular's built-in form arrays.
Adding Dynamic Form Fields
Let's say we want to add additional email input fields when the user clicks on a button. Update your template as follows:
<div formArrayName="emails">
<div *ngFor="let email of emails.controls; let i = index">
<input type="email" [formControlName]="i">
</div>
</div>
<button (click)="addEmail()">Add Email</button>
In your component, update the form group definition to include the "emails" form array:
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', Validators.required],
message: [''],
gender: [''],
emails: this.formBuilder.array([])
});
}
get emails() {
return this.myForm.get('emails') as FormArray;
}
addEmail() {
this.emails.push(this.formBuilder.control(''));
}
In the code above, we have added the "emails" form array to the form group. We have also defined helper methods to access and manipulate the form array. The addEmail()
method is used to add a new email field to the form array when the button is clicked.
Form Validation
Validating user input is a crucial part of form handling. Angular provides a wide range of validation options, including required fields, minimum and maximum lengths, custom validators, and more.
To add validation to a form field, you can use Angular's built-in validators or create custom validators. Let's see how to use required and minimum length validators.
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', [Validators.required, Validators.minLength(3)]],
message: ['', Validators.required],
gender: [''],
emails: this.formBuilder.array([])
});
}
In the code above, we have added two validators to the "name" field: Validators.required
makes the field required, and Validators.minLength(3)
sets the minimum length of the field to 3 characters. If the user enters a name less than 3 characters long, an error message will be displayed.
Submitting the Form
Once the user has filled out the form, we need to handle the form submission. In our onSubmit()
method, we can perform tasks like data validation, form submission to an API, or navigating to a different page. For now, let's log the form data to the console:
onSubmit() {
if (this.myForm.valid) {
console.log(this.myForm.value);
}
}
Make sure to add the following line of code to your template to bind the submit event to the onSubmit()
method:
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
That's it! You have now learned how to build dynamic and responsive user inputs in Angular. Forms are a fundamental part of web development, and Angular provides an efficient and powerful way to handle them. Feel free to explore more advanced topics and features of Angular forms, such as form validation, form arrays, form builders, and custom validators. Happy coding!
Conclusion
In this tutorial, we explored the world of Angular forms and learned how to build dynamic and responsive user inputs using Reactive forms. We walked through the process of setting up a project, creating a form, binding to form controls, adding dynamic form fields, and implementing form validation. With this knowledge, you can now create powerful and interactive forms in your Angular applications. Have fun experimenting with different form controls and validation options to create even more engaging user experiences.
Happy coding!