Angular Error Handling: Dealing with Errors in Angular Applications
"Error handling is essential in Angular applications. Learn how to handle compile-time and runtime errors effectively, provide meaningful error messages, and test your error handling logic."
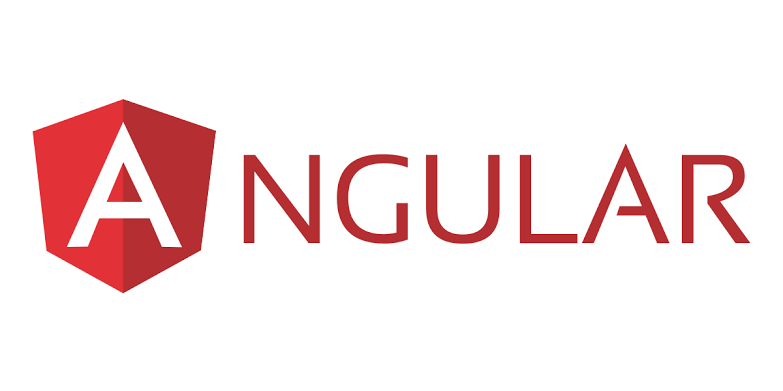
Angular Error Handling: Dealing with Errors in Angular Applications
Developing Angular applications involves dealing with various types of errors that can occur during runtime. Proper error handling is essential to ensure smooth application execution and provide a good user experience.
In this blog post, we will explore different techniques and best practices for handling errors in Angular applications. Let's dive in!
1. Error Handling Overview
Errors in Angular applications can be classified into two main categories:
- Compile-time errors: These errors occur during the compilation phase and prevent the application from being built successfully. Common examples include syntax errors, missing imports, and undefined variables.
- Runtime errors: These errors occur during the execution phase of the application. They can be caused by various factors such as network issues, server-side errors, and client-side validation failures.
Both types of errors need to be handled properly to maintain application stability and provide meaningful feedback to users.
2. Dealing with Compile-Time Errors
The Angular CLI provides a built-in mechanism to handle compile-time errors. When a compile-time error occurs, the CLI displays an error message along with the line number and file name where the error occurred. It also prevents the application from being built until the error is resolved.
To handle compile-time errors effectively, follow these best practices:
- Use a code editor with syntax highlighting and error checking: Modern code editors like Visual Studio Code provide real-time syntax highlighting and error checking. This helps you identify and fix syntax errors quickly.
- Pay attention to build output: During a build, the Angular CLI generates a build output that includes information about any compile-time errors. Make sure to review this output and resolve any issues before deploying the application.
- Utilize linting tools: Linting tools like ESLint and TSLint can catch potential syntax errors and coding issues. Integrate these tools into your development workflow to catch errors early.
3. Handling Runtime Errors
Unlike compile-time errors, runtime errors can occur during the execution phase of your application. These errors can lead to application crashes or unexpected behavior, making proper error handling crucial.
Here are some techniques to effectively handle runtime errors in Angular:
- Try-catch blocks: Use JavaScript's try-catch blocks to catch and handle synchronous errors. Wrap potentially error-prone code within a try block, and catch any exceptions that occur. You can then display an appropriate error message to the user or log the error for further analysis.
- Error handlers: Angular provides a global error handler mechanism that allows you to handle uncaught errors in a centralized manner. Implement the
ErrorHandler
interface to create a custom error handler that suits your application's needs. - Reacting to HTTP errors: When making HTTP requests in Angular, errors can occur due to network issues, server-side errors, or invalid responses. Use the
catchError
operator from therxjs
library to handle HTTP errors in a functional, declarative manner.
4. Providing Meaningful Error Messages
Error messages play a crucial role in user experience. A good error message should be clear, concise, and provide meaningful information about the error. It should also suggest potential solutions or actions the user can take to resolve the error.
When handling errors in your Angular application, make sure to:
- Display user-friendly error messages: Avoid showing technical error details directly to users. Instead, provide a friendly message that explains the issue in simple terms.
- Include error codes or identifiers: If applicable, include error codes or identifiers in the error message. This can help support teams troubleshoot and debug issues more effectively.
- Log error details: Capture and log error details in your application's logging mechanism. This allows developers and support teams to analyze errors and detect patterns.
5. Testing Error Handling
As with any other feature of your application, error handling should be tested thoroughly. Here are some tips to effectively test your error handling logic:
- Write unit tests: Write unit tests for individual error handling functions and code paths. Ensure that each function behaves as expected when encountering different types of errors.
- Simulate error scenarios: Use testing techniques to simulate specific error scenarios such as network failures, server errors, or invalid responses. Verify that your application handles these scenarios gracefully.
- Test error messages: Evaluate error messages displayed to users. Check their accuracy, clarity, and correctness. Consider edge cases and unusual scenarios to ensure your error messages cover all potential errors.
6. Summary
Proper error handling is crucial for building robust and user-friendly Angular applications. By following best practices, utilizing Angular's error handling mechanisms, and providing meaningful error messages, you can ensure a smooth and error-free user experience.
Remember to thoroughly test your error handling logic to catch any potential issues and ensure that your application behaves as expected in different error scenarios.
Keep learning and exploring Angular's error handling features to become a more proficient Angular developer!