Angular Directives: A Deep Dive into Angular's Powerful Feature
Angular directives allow you to extend HTML with custom behaviors. Learn about component, attribute, and structural directives and their use cases in this comprehensive guide. Enhance your Angular projects with powerful directives!
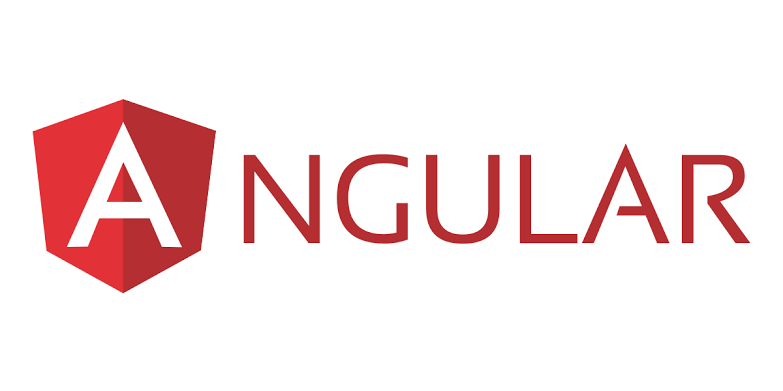
Introduction
Angular is a popular JavaScript framework for building robust and dynamic web applications. One of its most powerful features is directives, which allow you to extend HTML with custom behaviors and create reusable components. In this article, we will take a deep dive into Angular directives and explore their various types and use cases. By the end, you'll have a solid understanding of how directives work and how to leverage them in your Angular projects.
What Are Angular Directives?
Angular directives are markers on a DOM element that tell Angular to attach a specific behavior or functionality to that element. They enhance the HTML syntax by allowing you to extend it with custom elements, attributes, and structural directives. Angular provides a set of built-in directives, and you can also create your own custom directives.
Types of Angular Directives
Angular directives can be grouped into three main categories:
- Components: These are directives with a template. They represent reusable user interface components and encapsulate their behavior and presentation logic.
- Attribute Directives: These change the appearance or behavior of a DOM element by applying a specific behavior to an existing HTML element. They are represented as attributes on HTML elements.
- Structural Directives: These change the structure of the DOM by adding or removing elements. They are represented as attributes on HTML elements and use a special syntax to denote their behavior.
Creating and Using Directives
In Angular, you can create your own custom directives to extend the functionality of HTML elements or create reusable components. Here's how you can create and use directives:
1. Component Directives
To create a component directive, you need to use the @Component
decorator provided by Angular. The decorator allows you to specify the selector, template, and other properties of the component. Here's an example:
@Component({
selector: 'app-custom-component',
template: '<h1>I am a custom component</h1>',
})
export class CustomComponent {
// Component logic goes here
}
To use the component directive in your HTML template, you simply add the selector as an HTML element. For example:
<app-custom-component></app-custom-component>
2. Attribute Directives
Creating an attribute directive is similar to creating a component directive. You use the @Directive
decorator and specify the selector in the form of an attribute. Here's an example:
@Directive({
selector: '[appCustomDirective]'
})
export class CustomDirective {
// Directive logic goes here
}
To use the attribute directive, you simply add the directive's selector as an attribute on an HTML element. For example:
<div appCustomDirective>This div will have the custom directive applied</div>
3. Structural Directives
Structural directives provide a way to conditionally manipulate the DOM structure. Angular provides two built-in structural directives: *ngIf
and *ngFor
. Here's an example of how to use these directives:
<div *ngIf="showDiv">This div is conditionally rendered</div>
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
In the above example, the *ngIf
directive conditionally renders the div based on the value of the showDiv
property. The *ngFor
directive generates multiple list items based on the items
array.
Directive Lifecycle Hooks
Angular provides a set of lifecycle hooks that allow you to tap into specific moments in the lifecycle of a directive. These hooks enable you to perform actions at various stages, such as initialization, change detection, and destruction. Some of the commonly used lifecycle hooks for directives include:
ngOnInit
: Called after the directive's data-bound properties have been initialized.ngOnChanges
: Called whenever the directive's input properties change.ngOnDestroy
: Called right before the directive is destroyed.
Conclusion
Angular directives are a powerful feature that allows you to extend HTML and create reusable components. By utilizing component, attribute, and structural directives, you can enhance the behavior and appearance of your HTML elements. Additionally, you can create your own custom directives to encapsulate specific behaviors or encapsulate reusable UI components.
By understanding the different types of directives and their use cases, you'll have the tools to build more modular and maintainable Angular applications. Start leveraging the power of directives in your projects and take your Angular skills to the next level!