Angular Components: Exploring the Building Blocks of Angular
Learn how to create and use components in Angular! Discover the power of encapsulating UI logic, component communication, and building interactive user interfaces. A beginner-friendly guide to mastering Angular components.
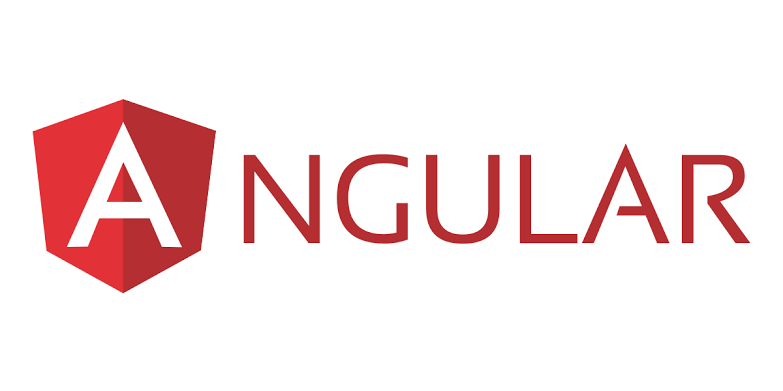
Introduction
Welcome to our beginner-friendly guide on Angular components! In this article, we will explore the building blocks of Angular and learn how to create and use components effectively. Angular components are the fundamental building blocks of Angular applications, and understanding them is essential for successful Angular development. So let's dive into the world of Angular components and uncover their power!
What are Components?
In Angular, components are the basic user interface (UI) elements that make up an application. They encapsulate the UI logic and provide a reusable and modular way to build user interfaces. Components consist of three key components:
- Template: The HTML markup that determines the structure and layout of the component.
- Class: The TypeScript code that defines the component's behavior and properties.
- Metadata: Additional configuration that specifies how the component should be processed and used.
By combining these three components, we can create powerful and interactive UI elements in Angular.
Creating a Component
Creating a component in Angular is a straightforward process. Let's walk through the steps involved:
Step 1: Generate a Component
To generate a new component, we can use the Angular CLI (Command Line Interface) command ng generate component
or its shorthand ng g c
. This command creates all the necessary files and folders for the component, including the template, class, and metadata.
$ ng generate component my-component
This command will create a new folder called my-component
with the necessary files.
Step 2: Configure the Component
Once the component is generated, we can configure its template, class, and metadata.
Template
The template file resides in the component folder and has a file extension of .html
. This is where we define the structure and layout of the component using HTML markup. We can also use Angular directives and bindings to make the template dynamic and interactive.
Class
The class file resides in the component folder and has a file extension of .ts
. This is where we define the component's behavior and properties using TypeScript code. We can define properties, write methods, and handle events within the class file.
Metadata
The metadata is defined using a decorator, which is a special kind of TypeScript declaration. The decorator contains essential configuration details for the component, such as the selector, template URL, and style URLs. The metadata is defined at the top of the class file using the @Component
decorator.
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponent {
// Component logic goes here
}
Step 3: Use the Component
Once the component is created and configured, it can be used in other parts of the application. To use a component, we need to include its selector in the HTML markup of another component. Angular will then render the component and replace its selector with the generated HTML markup.
<app-my-component></app-my-component>
By following these simple steps, we can create and use components in our Angular applications.
Component Communication
Component communication is an essential concept in Angular applications. There are two main ways components can communicate with each other:
1. Input and Output Properties
Components can pass data to child components using input properties, and child components can emit events back to the parent component using output properties. By using input and output properties, components can exchange data and trigger actions based on user interactions or application events.
// Parent Component
<app-child [greeting]="parentGreeting" (buttonClick)="handleButtonClick($event)"></app-child>
// Child Component
@Component({
...
})
export class ChildComponent {
@Input() greeting: string;
@Output() buttonClick: EventEmitter<string> = new EventEmitter<string>();
handleClick() {
this.buttonClick.emit('Button clicked!');
}
}
In the example above, the parent component passes the parentGreeting
value to the child component using the [greeting]
input property binding. The child component, in turn, emits an event using the (buttonClick)
output property and passes the event data back to the parent component.
2. Service Communication
Components can also communicate with each other using shared services. A service is a class that can be injected into multiple components, allowing data and functionality to be shared across components. By using services, we can establish a centralized communication channel between components.
// Shared Service
@Injectable({
providedIn: 'root'
})
export class DataService {
private dataSubject: BehaviorSubject<string> = new BehaviorSubject<string>('');
getData(): Observable<string> {
return this.dataSubject.asObservable();
}
setData(data: string) {
this.dataSubject.next(data);
}
}
// Component A
export class ComponentA {
constructor(private dataService: DataService) {}
setData(data: string) {
this.dataService.setData(data);
}
}
// Component B
export class ComponentB {
data: string;
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getData().subscribe((data: string) => {
this.data = data;
});
}
}
In the example above, we have a shared DataService
that exposes a data subject and its corresponding observable. ComponentA
sets the data using the setData
method, and ComponentB
subscribes to the observable to receive the data updates.
Conclusion
Understanding Angular components is essential for building robust and modular Angular applications. By creating and configuring components, and by leveraging component communication techniques, we can create interactive and reusable user interfaces.
Now that you have a solid understanding of Angular components, try creating your own components and experiment with different communication patterns. The more you practice, the more proficient you will become with Angular.
Happy coding!