Angular and Firebase: Creating Real-Time Web Applications
Learn how to build real-time web applications using Angular and Firebase. Discover the power of real-time data synchronization and create dynamic and responsive apps with ease.
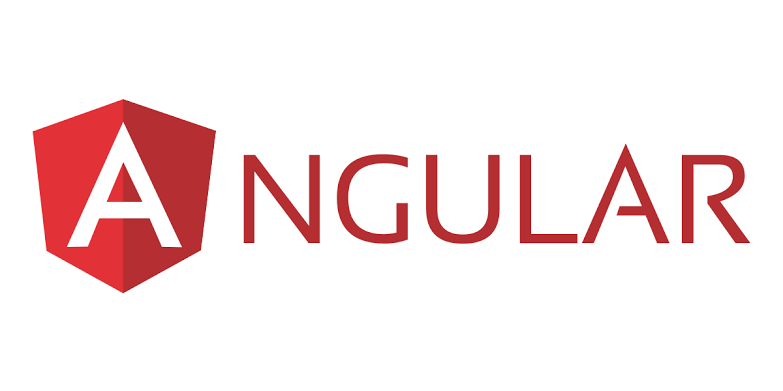
Angular and Firebase: Creating Real-Time Web Applications
Are you looking to build a real-time web application? Look no further! In this tutorial, we'll explore how to leverage the power of Angular and Firebase to create dynamic and responsive web applications that update in real-time.
Introduction to Angular and Firebase
Angular is a powerful and popular JavaScript framework that enables developers to create single-page applications with ease. It provides a robust set of tools and features, including two-way data binding, dependency injection, and a component-based architecture.
Firebase is a comprehensive cloud-based platform offered by Google that provides a range of services for building web and mobile applications. It includes services such as real-time database, authentication, hosting, and cloud functions.
Combining Angular and Firebase allows developers to create scalable and real-time web applications without worrying about server infrastructure or complex backend logic. Let's dive into the world of real-time web applications with Angular and Firebase!
Setting up your Angular Project
Before we get started, make sure you have Node.js and Angular CLI installed on your machine. If you don't have them installed, follow the official documentation to install them.
To create a new Angular project, open your command prompt or terminal and run the following command:
ng new my-realtime-app
This command will create a new Angular project named "my-realtime-app".
Navigate to the project directory by running the following command:
cd my-realtime-app
Now, let's install the dependencies for Firebase by running the following command:
npm install firebase @angular/fire
This command will install the necessary packages for Firebase integration with Angular.
Setting up Firebase
To use Firebase in your Angular project, you'll need to create a Firebase project and obtain the configuration details. Follow these steps to set up Firebase:
- Go to the Firebase console and create a new project.
- Click on "Add app" and select the "Web" option.
- Provide a name for your app and click on the "Register app" button.
- Copy the configuration details, including the API key, project ID, and other information. We'll need these details later.
Now that you have set up your Firebase project, let's integrate it with your Angular application.
Integrating Firebase with Angular
In your Angular project, open the src/environments/environment.ts
file and add the Firebase configuration details you copied earlier:
export const environment = {
production: false,
firebaseConfig: {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
}
};
Replace the placeholder values with the corresponding values from your Firebase project.
Next, open the src/app/app.module.ts
file and add the necessary imports to integrate Firebase with your Angular application:
import { AngularFireModule } from '@angular/fire';
import { AngularFireDatabaseModule } from '@angular/fire/database';
import { environment } from '../environments/environment';
Make sure to add these imports to the imports
array in the @NgModule
decorator:
@NgModule({
declarations: [
// Component declarations
],
imports: [
BrowserModule,
AngularFireModule.initializeApp(environment.firebaseConfig),
AngularFireDatabaseModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
With these steps, you have successfully integrated Firebase with your Angular project.
Creating a Real-Time Database
Now that your Angular project is set up and Firebase is integrated, let's create a real-time database. The Firebase Realtime Database is a NoSQL cloud-hosted database that allows data synchronization in real-time.
Open the src/app/app.component.ts
file and import the necessary dependencies:
import { Component } from '@angular/core';
import { AngularFireDatabase } from '@angular/fire/database';
Next, add the necessary code to the AppComponent
class to create a reference to the database and retrieve data:
@Component({
selector: 'app-root',
template: `
<h1>Real-Time Data</h1>
<ul>
<li *ngFor="let item of items | async">{{ item.value }}</li>
</ul>
`
})
export class AppComponent {
items: any;
constructor(private db: AngularFireDatabase) {
this.items = db.list('items').valueChanges();
}
}
In the above code, we import the Component
and AngularFireDatabase
dependencies. We then declare a class named AppComponent
and create a reference to the database using AfDatabase.list('items')
. We assign the resulting data to the items
variable and display it using Angular's template syntax.
Displaying Real-Time Data
To display real-time data in your Angular application, open the src/app/app.component.html
file and add the following code:
<h1>Real-Time Data</h1>
<ul>
<li *ngFor="let item of items | async">{{ item.value }}</li>
</ul>
The above code uses {{ item.value }}
to display the value of each item in the items
array. The async
pipe allows Angular to handle the observable and update the UI automatically whenever new data is added to the database.
Testing Your Real-Time Application
To test your real-time application, open your command prompt or terminal, navigate to your project directory, and run the following command:
ng serve
This command will start the local development server, and your application will be accessible at http://localhost:4200
.
Open your browser and visit http://localhost:4200
. You should see the "Real-Time Data" heading and a list of items. If you add, edit, or delete items from the Firebase console, your Angular application will update in real-time!
Conclusion
Congratulations! You've successfully created a real-time web application using Angular and Firebase. You've learned how to set up an Angular project, integrate Firebase, create a real-time database, and display real-time data in your application.
Real-time web applications offer a dynamic and interactive user experience, and Angular and Firebase provide an excellent platform to build them rapidly. With the power of Angular's component-based architecture and Firebase's real-time database, you can create scalable and responsive web applications with ease.
Take your application to the next level by exploring additional Firebase services such as authentication, hosting, and cloud functions. Happy coding!